Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial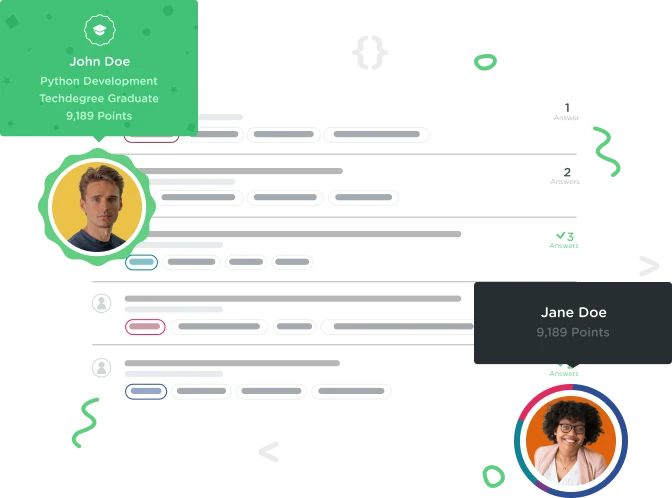

Jason Peay
16,515 PointsHow to use.On()
On line 6 of app.js, fix the code so when you add a color li dynamically, the click handler will still work.
I am not sure how to complete this task. I followed the code I wrote in the app.js when I watched the video, but it isn't working. Am I missing something or doing something wrong.
//Problem: No user interaction causes no change to application
//Solution: When user interacts cause changes appropriately
var color = $(".selected").css("background-color");
//When clicking on control list items
$(".controls").click, (function(){
//Deselect sibling elements
$(this).siblings().removeClass("selected");
//Select clicked element
$(this).addClass("selected");
//cache current color
color = $(this).css("background-color");
});
//When "New Color" is pressed
$("#revealColorSelect").click(function(){
//Show color select or hide the color select
changeColor();
$("#colorSelect").toggle();
});
//update the new color span
function changeColor() {
var r = $("#red").val();
var g = $("#green").val();
var b = $("#blue").val();
$("#newColor").css("background-color", "rgb(" + r + "," + g +", " + b + ")");
}
//When color sliders change
$("input[type=range]").change(changeColor);
//When "Add Color" is pressed
$("#addNewColor").click(function(){
//Append the color to the controls ul
var $newColor = $("<li></li>");
$newColor.css("background-color", $("#newColor").css("background-color"));
$(".controls ul").append($newColor);
//Select the new color
$newColor.click();
});
//On mouse events on the canvas
//Draw lines
<!DOCTYPE html>
<html>
<head>
<title>Simple Drawing Application</title>
<link rel="stylesheet" href="css/style.css" type="text/css" media="screen" title="no title" charset="utf-8">
</head>
<body>
<canvas width="600" height="400"></canvas>
<div class="controls">
<ul>
<li class="red selected"></li>
<li class="blue"></li>
<li class="yellow"></li>
</ul>
<button id="revealColorSelect">New Color</button>
<div id="colorSelect">
<span id="newColor"></span>
<div class="sliders">
<p>
<label for="red">Red</label>
<input id="red" name="red" type="range" min=0 max=255 value=0>
</p>
<p>
<label for="green">Green</label>
<input id="green" name="green" type="range" min=0 max=255 value=0>
</p>
<p>
<label for="blue">Blue</label>
<input id="blue" name="blue" type="range" min=0 max=255 value=0>
</p>
</div>
<div>
<button id="addNewColor">Add Color</button>
</div>
</div>
</div>
<script src="//code.jquery.com/jquery-1.11.0.min.js" type="text/javascript" charset="utf-8"></script>
<script src="js/app.js" type="text/javascript" charset="utf-8"></script>
</body>
</html>
2 Answers
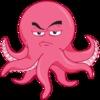
Kieran Black
9,139 PointsHi Jason,
Line 6 of the .js file as you correctly point out is incorrect. The challenge wants you to to use the jQuery on() method.
If we look at the second example in the documentation we can see:
$( "#dataTable tbody" ).on( "click", "tr", function() {
console.log( $( this ).text() );
});
This gives us the format of what is expected for this method, if we apply it to our situation we end up with:
$(".controls").on("click", "li", function(){...});
The full example is here:
//Problem: No user interaction causes no change to application
//Solution: When user interacts cause changes appropriately
var color = $(".selected").css("background-color");
//When clicking on control list item
$(".controls").on("click", "li", function(){
//Deselect sibling elements
$(this).siblings().removeClass("selected");
//Select clicked element
$(this).addClass("selected");
//cache current color
color = $(this).css("background-color");
});
//When "New Color" is pressed
$("#revealColorSelect").click(function(){
//Show color select or hide the color select
changeColor();
$("#colorSelect").toggle();
});
//update the new color span
function changeColor() {
var r = $("#red").val();
var g = $("#green").val();
var b = $("#blue").val();
$("#newColor").css("background-color", "rgb(" + r + "," + g +", " + b + ")");
}
//When color sliders change
$("input[type=range]").change(changeColor);
//When "Add Color" is pressed
$("#addNewColor").click(function(){
//Append the color to the controls ul
var $newColor = $("<li></li>");
$newColor.css("background-color", $("#newColor").css("background-color"));
$(".controls ul").append($newColor);
//Select the new color
$newColor.click();
});
//On mouse events on the canvas
//Draw lines
Hope this helps.
KB

Aaron Martone
3,290 PointsYou can lookup jQuery's .on()
documentation online.
Simply put:
$(<selector>).on(<event>, <handler>);
That's the simplest implementation. It does support other syntaxes that allow you to use delegation.