Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial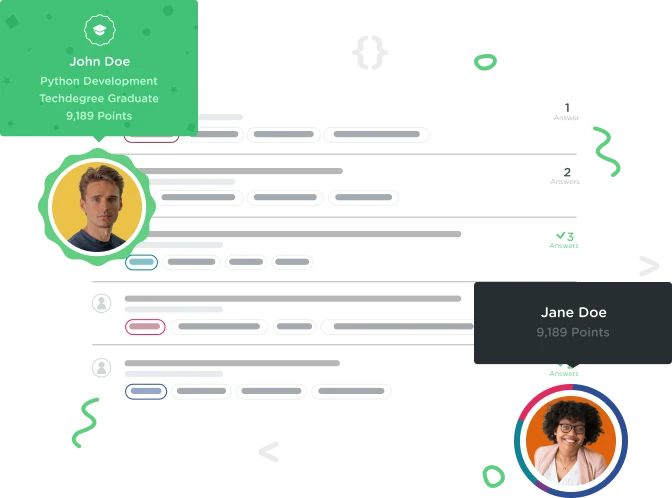
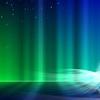
karthikkn
5,397 PointsHow to verify password_verify function in login form
Registration page
<?php require_once 'includes/session.php';?>
<?php require_once 'includes/db.php'; ?>
<?php require_once 'functions.php'; ?>
<?php
if (isset($_POST['submit'])) {
$required_field = array("email", "password", "firstname", "lastname");
validate_presences($required_field);
if (empty($errors)) {
$email = mysqli_real_escape_string($connection, $_POST['email']);
$password = password_hash($_POST['password'], PASSWORD_BCRYPT);
$firstname = mysqli_real_escape_string($connection, $_POST['firstname']);
$lastname = mysqli_real_escape_string($connection, $_POST['lastname']);
$query = "INSERT INTO users (email, password, firstname, lastname) VALUES ('$email', '$password', '$firstname', '$lastname')";
$result = mysqli_query($connection, $query);
if (!$result) {
echo "Email already exists";
} else {
echo 'pass';
}
}
}
?>
<!DOCTYPE html>
<html>
<head>
<title>hillspeak</title>
</head>
<body>
<h2>Register here</h2>
<?php echo form_errors($errors); ?>
<form action="" method="post">
Email: <input type="email" name="email"><br>
Password: <input type="password" name="password"><br>
First Name: <input type="text" name="firstname"><br>
Last Name: <input type="text" name="lastname"><br>
<input type="submit" name="submit" value="register">
</form>
</body>
</html>
Login page
<?php require_once 'includes/session.php';?>
<?php require_once 'includes/db.php'; ?>
<?php require_once 'functions.php'; ?>
<?php
if (isset($_POST['submit'])) {
$required_fields = array("email", "password");
validate_presences($required_fields);
if (empty($errors)) {
$email = mysqli_real_escape_string($connection, $_POST['email']);
$password = $_POST['password'];
$query = "SELECT * FROM users WHERE email = '$email' AND password = '$password'";
$result = $connection->query($query);
$row = mysqli_num_rows($result);
if ($row == 1) {
echo 'found';
} else {
echo "not found";
}
}
}
?>
<!DOCTYPE html>
<html>
<head>
<title>hillspeak</title>
</head>
<body>
<h2>Log In</h2>
<?php echo form_errors($errors); ?>
<form action="" method="post">
Email: <input type="text" name="email"><br>
Password: <input type="password" name="password"><br>
<input type="submit" name="submit" value="Login">
</form>
<a href="register.php">Register</a>
</body>
</html>
3 Answers
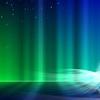
karthikkn
5,397 Pointshi Stefan Hoffmann, Thanks for reply i have made email field unique in database, I have found the problem, I will try to find a solution to that problem, Thanks for reply.
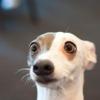
Stefan Hoffmann
24,811 PointsHello again,
another thing:
$password = $_POST['password'];
$query = "SELECT * FROM users WHERE email = '$email' AND password = '$password'";
You are open to SQL Injection at this point, because you do not escape $password properly. One could easily use " ' OR 1=1 OR ' "AS Password to log in as every user whose emailadress is known.
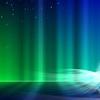
karthikkn
5,397 PointsHi, thanks for your help i have changed my login password field to $_POST['password'] to mysqli_real_escape_string($connection, $_POST['password']);
Stefan Hoffmann
24,811 PointsStefan Hoffmann
24,811 PointsHello !
Your question is not clear. Anyway, in your registration form there is an error. You insert a new user even after finding out, that a user with that email address already exists.
Depending on your mysql configuration you may have a problem in your password check. You can try this out by entering your password in different upper- and lowercase alternatives. You'll see that 'PASSword' = 'passWORD' in mysql.
To find out more and how to get around this problem, look it up here: https://dev.mysql.com/doc/refman/5.0/en/charset-binary-op.html
Greetings, Stefan