Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial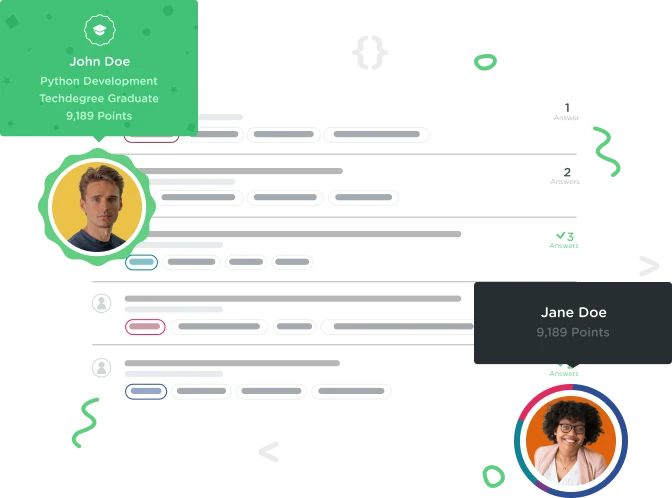

Sahil Kapoor
8,932 PointsHow to wait until a request is complete in node.js?
let request = https.get(`https://teamtreehouse.com/${username}.json`, response => {
console.log(response.headers);
});
In the above code I'm just making a get request to teamtreehouse API but the problem is that as JavaScript being Asynchronous the code after this runs first while the request is in process. So how do I make JavaScript synchronous so that it completes this process first and then do interpret further code.
2 Answers

Samuel Ferree
31,722 PointsMy google search indicates the best answer is this node package: https://www.npmjs.com/package/sync-request
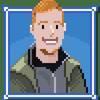
Balázs Buri
8,799 Pointsif you'd like to solve this without using an extra package you can refer to the nodejs documentation
this is the example code from the documentation with my comments:
const https = require('https');
https.get('https://encrypted.google.com/', (res) => { // <- this is a function that is called when there's a response. Waiting for a response is as easy as writing code inside this function (or use async await)
console.log('statusCode:', res.statusCode);
console.log('headers:', res.headers);
res.on('data', (d) => { //this is a function attached to the response's 'data' event. it's called every time a chunk of data arrives. (multiple times per request)
process.stdout.write(d);
});
}).on('error', (e) => { //the https.get function returns a request that can emit an error event. this is an eventlistener for that. try an invalid url to test this branch of your code
console.error(e);
});
hope this helps