Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial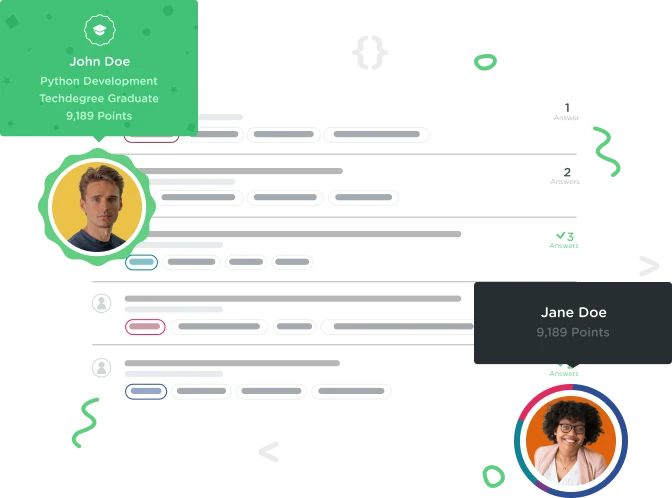

vikrant kaushal
Front End Web Development Techdegree Student 2,558 Pointshow to would i add user input and still run this code?
gill is console logging it and assigning a variable but what if i want the user to input the information
3 Answers
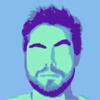
Cameron Childres
11,820 PointsHi Vikrant,
A simple way to do this would be using prompt()
, much like in the previous video:
const weather = prompt('What is the weather?');
With the code as written in the video the only acceptable answers will be 'sun', 'rain', or 'snow'. Otherwise the code will run the final else
statement.
If you want to view the response outside the console you could replace console.log()
with alert()
.
These methods work fine for testing but they aren't the most elegant ways for users to interact with a site. Stick with the courses and you'll learn more ways of receiving user inputs and displaying information, such as through text/dropdowns and changing HTML on the page with JavaScript. I hope this helps!

vikrant kaushal
Front End Web Development Techdegree Student 2,558 PointsThank you but how would we write the if else or it would be written still the same?
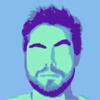
Cameron Childres
11,820 PointsThe logic stays the same so you wouldn't need to do anything besides swap out console.log()
for alert()
. All that you're doing is changing where the message is displayed. Like this:
const weather = prompt("What's the weather?");
if ( weather === 'sun' ) {
alert("It's sunny, so I'm going swimming.");
} else if ( weather === 'rain' ) {
alert("It's raining, so I will read a book.");
} else if ( weather === 'snow' ) {
alert("It's snowing, so I'm going sledding.");
} else {
alert("I don't know what I'm doing today.");
}
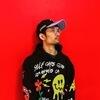
Paul Mamac
Full Stack JavaScript Techdegree Student 2,622 Pointsconst weather = prompt("What's the weather?");
if ( weather.toUpperCase === 'sun' ) {
alert("It's sunny, so I'm going swimming.");
} else if ( weather.toUpperCase === 'rain' ) {
alert("It's raining, so I will read a book.");
} else if ( weather.toUpperCase === 'snow' ) {
alert("It's snowing, so I'm going sledding.");
} else {
alert("I don't know what I'm doing today.");
}
This will make sure that even if they type the weather in uppercase it will still give the expected behavior.