Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial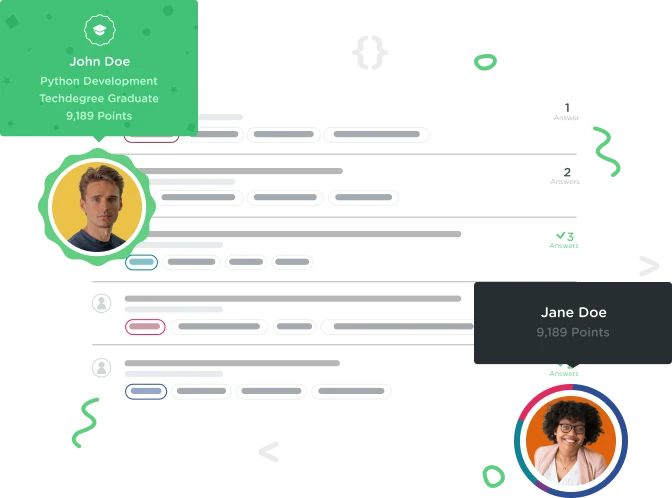
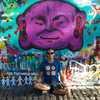
Andrew Stelmach
12,583 PointsHow to write your own 'action'
('handcode' branch) https://github.com/Yorkshireman/sebcoles/tree/handcode
I have a User model, and two models that inherit from that: Teacher and Student. They also have their own controllers that inherit from the User controller.
I also have a Group model.
group belongs to teacher, teacher has many groups. group has and belongs to many students. student has and belongs to many groups.
There is a join table for the HABTM relationships, called 'groups_students'.
I have managed to create a form element that allows me to set the 'type' of User to 'Student' or 'Teacher'.
The difficulty I have now is assigning students to groups in the view. I can do this in the console no problem, as outlined here: http://stackoverflow.com/questions/29223974/rails-4-habtm-how-to-set-multiple-ids-in-console/29227188#29227188
I'm stumped as to how to do this in a view. I want to do it in the Student's show view.
Can someone at least give me some guidance? I have a pretty good book on Rails, but I need to know roughly what I have to do.
2 Answers
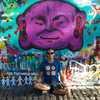
Andrew Stelmach
12,583 PointsIn the view:
<%= form_tag assign_to_group_path do %>
<%= hidden_field_tag :user_id, @user.id %>
<%= select_tag :group_id, options_from_collection_for_select(Group.all, "id", "title"), include_blank: true %>
<%= submit_tag "Assign to Class" %>
<% end %>
private part of users_controller:
def user_params
params[:user].permit(:type) if params[:user] # The 'if params[:user]' means an incomplete User object won't be rejected.
end
students_controller (inherits from users_controller):
def assign_to_group
@user=User.find(params[:user_id])
@group=Group.find(params[:group_id])
@user.groups << @group unless @user.groups.include? @group
@user.save!
redirect_to user_path @user
end
routes.rb:
post 'assign_to_group' => 'students#assign_to_group'
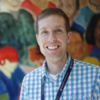
Brandon Barrette
20,485 PointsI would use checkboxes on the group form so you could check off the students you want in the group.
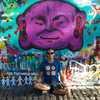
Andrew Stelmach
12,583 PointsThanks, I've seen that before, but I really would prefer to use a dropdown menu select field. I have that, but submit doesn't work properly.
I'm having a bash at using simple_form (see the 'simple_form' branch), but I'm getting a Nil class error when I submit (to do with the 'private' params.permit part in the controller.