Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial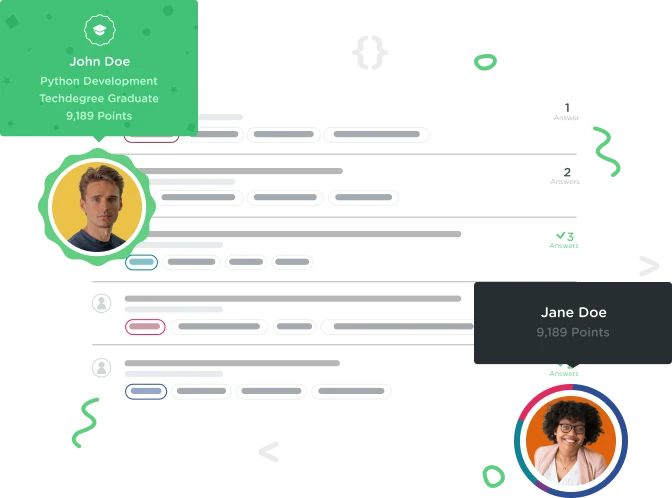

Benjamin Lim
2,747 PointsHow were we suppose to come to this conclusion with the information we've been given?
This is the correct answer below. I copied it from the forum, but i am absolutely stumped on how we were suppose to come to this conclusion with the information given? I re-watched the previous videos several times whilst concurrently referring to the apple Swift Programming Language book to try to pick up what to do here. I didn't even know we had to run the variable through the initialiser (did i miss this in the video? if so, please do tell where i can find it?). Furthermore, i didn't know we had to assign an integer value to run the code. Is it me? Or is this very demanding for an introduction. I completely understand that this is about problem solving, but i feel like I haven't received adequate guidance in recent lessons to perform the challenge tasks with confidence. Most of the challenge tasks before object oriented swift were very much possible but I've recently felt very lost and uninformed.
// Enter your code below
class Shape{
var numberOfSides: Int
init(sides numberOfSides: Int){
self.numberOfSides = numberOfSides
}
}
let someShape = Shape(sides: 3)
2 Answers
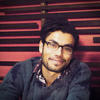
Dennis O'Keeffe
32,820 PointsHey Ben!
The challenge can come as tricky, but it is certainly important to get into the habit of learning about initialisers.
Most classes you may have dealt with may inherit their init method from a parent class, whereas classes that we create require some sort of init() method to initialise a class instance.
What you did was very useful for practising how to pass arguments through to the init method! Alternatively, the follow code also would have worked:
// Enter your code below
class Shape {
var numberOfSides:Int
init() {
//alternative to what you wrote, this will hardcode EVERY instance of Shape to have 4 sides
self.numberOfSides = 4
}
}
let someShape = Shape()
You can also have multiple init methods for different reasons! A great example in the Apple Documentation shows a struct for Celsius that has two init() methods which take arguments from Kelvin for one init() method and Fahrenheit for the other.
Check the docs out!
This phase of Swift took me ages to figure out, but just keep at it and refer to the docs for help and good practise and you'll be home and hosed!
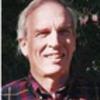
jcorum
71,830 PointsOK, step by step. First, we will create a class named Shape:
class Shape {
}
Then, inside the class, we will create a stored property named numberOfSides. Since we will not be assigning it a value we need to give it a type: Int:
class Shape {
var numberOfSides: Int
}
Since this is a class and not a struct, we need to write an initializer, as all stored properties must have a value (be initialized) when any Shape object is created. (Structs are different in that they have a default member-wise initializer "built-in".) Since we have only one stored property our initializer will have only one input parameter:
class Shape {
var numberOfSides: Int
init(sides numberOfSides: Int){
self.numberOfSides = numberOfSides
}
}
Here init acts like a function (or method). It takes a value when called and assigns that value to the stored property. Here the parameter has an internal name numberOfSides, an external name sides ,and a type Int. You need the keyword self here to disambiguate between the local variable the init will create to hold the numberOfSides value and the stored property which will be initialized with it. If you wrote it this way:
init(sides aNumberOfSides: Int){
numberOfSides = aNumberOfSides
}
you wouldn't need to use self, as their is no ambiguity between numberOfSides (the stored property) and the input parameter/local variable aNumberOfSides.
So now the class is done, and we want a Shape object:
let someShape = Shape(sides: 3)
Here we create a constant named someShape and assign it a Shape object with 3 sides. Note that it has Shape(sides: 3) rather than Shape(numberOfSides: 3). That's because, in the init(), you gave it an external parameter name. You now have a Shape object with 3 sides.
Hope this helps!