Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial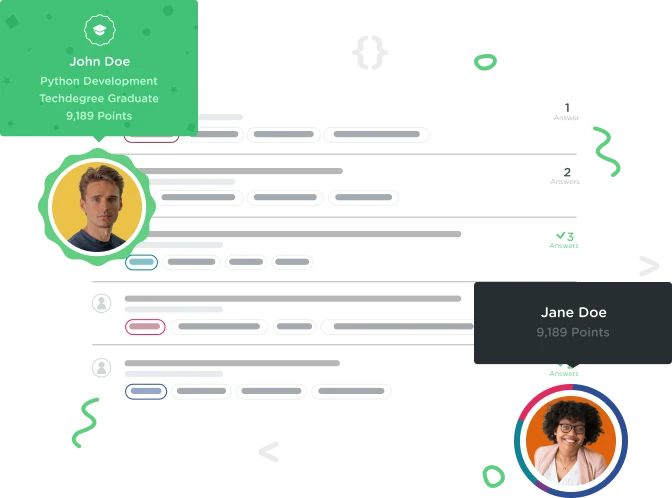
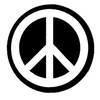
john larson
16,594 Pointshow would I access "keys" in this scenario? (using a for loop, not a for in)
//if this is the array
var family = [
{
title: "Derblin Prime",
position: "Matriarch",
name: "Dionne",
subjugation: "Supreme"
}
];
var html = "";
var i;
//and this is the loop
for(i = 0; i < family.length; i += 1){
html+= "<h3>" + family[i].title + "</h3>";
}
//I understand that family[i].title accesses the value
//how would I access the key?
3 Answers
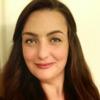
Jennifer Nordell
Treehouse TeacherYou could use this line to return an array of the keys.
console.log(Object.keys(family[0]));
Try that one and see if it gives you the output you're looking for!
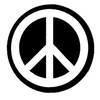
john larson
16,594 PointsThanks Jennifer, that outputs the information to the console. I'm trying to picture how I would incorporate that into the current code or is there a way to access both keys and values in an array/object combination and print to the page? Also that code looks like maybe a predefined function?
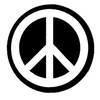
john larson
16,594 Pointsnever mind, I think I got it. Thank you so much. Still wondering about Object.keys though. If that's what it was meant to do or if it was kind of tweek or hack?
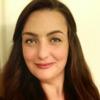
Jennifer Nordell
Treehouse Teacherjohn larson it's no hack. Here's the MDN documentation
https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Object/keys
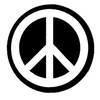
john larson
16,594 PointsI got it to do something pretty close to what I was looking for. I inserted as the top line in the loop so above each set of values it print on top, kinda like a header.
fun. Thanks again :D
var html = "";
var i;
for(i = 0; i < family.length; i += 1){
html+= Object.keys(family[0]) + "<br>"
+ "<h3>" + family[i].title + "</h3>"
+ "<p>" + family[i].position + "</p>"
+ "<p>" + family[i].name + "</p>"
+ "<p>" + family[i].subjugation + "</p>";
}
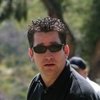
Mike LaPan
5,190 PointsI used this to access the individual titles. Is this right, or is there a better way?
var keys = Object.keys(family[0]);
console.log(keys[0]);
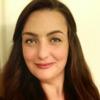
Jennifer Nordell
Treehouse TeacherI don't think so without using a for in
. Or if there is, I haven't come across it yet
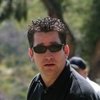
Mike LaPan
5,190 PointsLOL, it was within John's "for in" loop above. Yeah, without it I doubt it would work. :-)
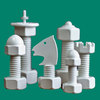
Steven Parker
230,274 PointsThis struck me as funny...
for(i = 0; i < family.length; i += 1){
html+= "<h3>" + family[i].title + "</h3>";
}
//I understand that family[i].title accesses the value
//how would I access the key?
john larson
16,594 Pointsjohn larson
16,594 PointsI get that the "for loop" is related to arrays, and the "for in" to objects. I'm just curious about the possibilities because in this video Dave was showing us the object within the array, but we only accessed the value, not the key.