Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial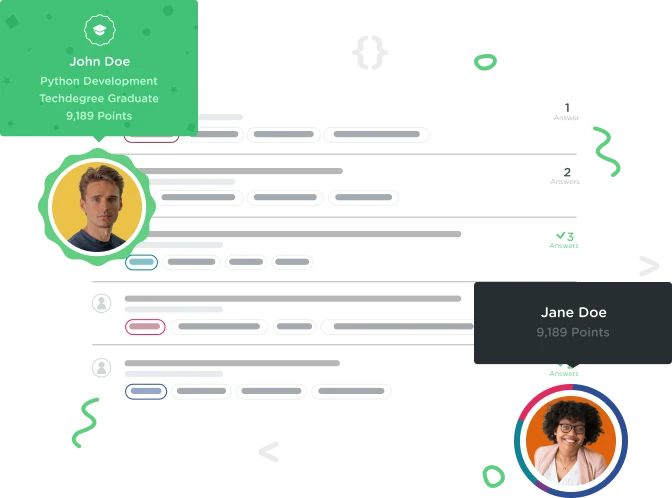

Michael Russell
1,575 PointsHow would I change the code to include the super() function?
I was able to successfully complete this Python Task Challenge with the following code, but I'm curious as to how I might change it to include the super() function. The instructions say "You'll probably need super() for this," but even with the previous videos/examples of how super() is used, I don't see how/why I could use/need it in this task. Could someone please explain it to me?
class Liar(list):
def __init__(self):
self.slots = []
def add(self, item):
self.slots.append(item)
def __len__(self):
return len(self.slots) + 2
1 Answer
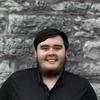
Michael Hulet
47,913 PointsThe super
function is necessary whenever you want to run the original implementation of a method you're overriding from the superclass. In this case, you'll need to call len
on super
to ensure that the number you return from your implementation of __len__
never matches the actual len
from the superclass
For example, you can manipulate an instance of your Liar
class to return the same length as its superclass by append
ing 2 items to it, like this:
example = Liar()
example.append("One")
example.append("Two")
In this case, calling len
on example
will return 2
, which is the right number. This happens because your add
function never gets called, so the len
of your slots
property will be 0
. Your implementation of __len__
returns the value of len(self.slots) + 2
, and 0 + 2
is 2
The best way to solve this challenge, guaranteeing that it will never return the proper value, would be to apply some arbitrary mathematical operation to another arbitrary number and the value of len(super())
. That way, you know the number you definitely can't return, and you're always applying an operation to it in order to guarantee that it changes, and you don't need to override __init__
or define any of your own methods or properties