Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial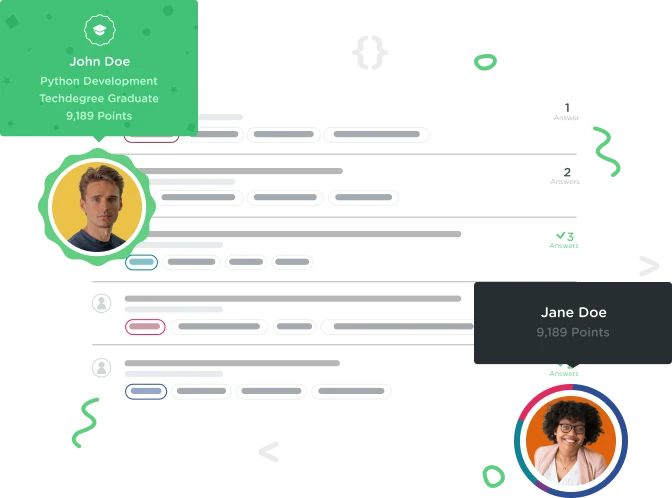

Jonathan Haro
18,101 PointsHow would I check to see if an email entered in a registration form was already in the a mongo database?
Would I add an 'else if' block after the 'if' block which confirms that the passwords entered at the form match?
else if (User.findOne({req.body.email}) == true ) {
var err = new Error('A user with that email has already registered. Please use a different email..')
err.status = 400;
return next(err);
}
pretty sure you can't use methods like
User.findOne({req.body.email})
within a route.
If that's the case then does this check happen in the user.js? Would I create another method off the UserSchema.statics like:
UserSchema.statics.emailCheck = function(email, callback) {
}
3 Answers

Aemiro Allison
480 PointsIf your User Schema has an email field then you can check the email field on the User model with the email entered from the form in req.body.email
//a simple if/else to check if email already exists in db
User.findOne({ email: req.body.email }, function(err, user) {
if(err) {
//handle error here
}
//if a user was found, that means the user's email matches the entered email
if (user) {
var err = new Error('A user with that email has already registered. Please use a different email..')
err.status = 400;
return next(err);
} else {
//code if no user with entered email was found
}
});

Seth Kroger
56,413 PointsAre you using Mongoose? Model.findOne() never returns the actual document, which would be a synchronous action. It either takes a callback that runs when the query is finished or returns a query if you didn't specify a callback which needs to be exec()ed with a callback or promise.

Aemiro Allison
480 PointsYou do not have access to the next() function when creating your User model, so you must return the error or user (either will work fine) to the callback. You also don't have access to the request object in your User model definition, so you need to replace 'req.body.email' with entered argument 'email'.
Also in your User model, if the email does not exist, all the code within the exec function's callback will be ignored and can lead to hidden errors. You should call the callback with the user to keep code exceution predictable if an email is found or not.
Lastly, if your going to use 'next(err)' to throw your errors then you should have an error handling express middleware to catch all your errors. (https://expressjs.com/en/guide/error-handling.html)
UserSchema.statics.emailCheck = function(email, callback) {
// replaced req.body.email with email
User.findOne({ email: email })
.exec(function (error, user) {
if (error) {
return callback(error);
}
// Pass user to callback and handle whether email exist in the callback.
callback(null, user);
});
};
User.emailCheck(req.body.email, function (error, user) {
if (error) {
return next(error);
}
if( user ) {
// Tell client that the email already exist.
res.status(401).json({error: 'User with email already found'});
} else {
// Code if no user with entered email was found.
res.json({ message: 'Successfully created account.'});
}
});

Jonathan Haro
18,101 PointsThanks!!
Jonathan Haro
18,101 PointsJonathan Haro
18,101 PointsOk so this is what I have in my User model:
Would I then add code in my index.js to run the above function?