Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial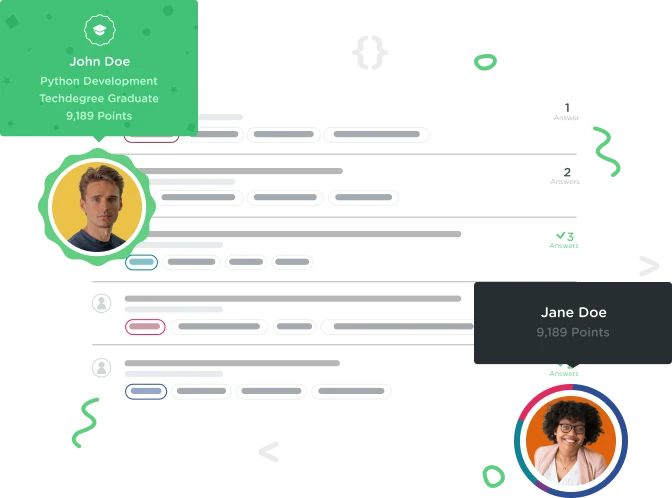

William J. Terrell
17,403 PointsHow would I clear the value of an input with jQuery?
First, I'm afraid I must start with a very long explanation. I apologize in advance for the weight of it. ^^;
I am working on a form for our University. This form has three fields and I have use jQuery to display additional fields based on the value of those fields:
One: Will you be an Equestrian student? (Yes / No) If "Yes", the following fields are displayed: "What seat will you ride?", "What time will you visit?".
Two: Would you like to meet with a Professor? (Yes / No) if "Yes", the following fields are displayed: "First Choice (by dept.)", "Second Choice (by dept.)".
Three: Are you interested in Athletics? (Yes / No) if "Yes", the following fields are displayed: "Sport Choice", "Would you like to meet with a Coach?".
The jQuery for this is as follows:
function hideEQSFields() {
$("#eqs_seat_row").hide();
$("#eqs_visit_time_row").hide();
}
function hideMajorFields() {
$("#firstChoice_row").hide();
$("#secondChoice_row").hide();
}
function hideAthleticFields() {
$("#sport_row").hide();
$("#meetCoach_row").hide();
}
function showEQSFields() {
$("#eqs_seat_row").show();
$("#eqs_visit_time_row").show();
}
function showMajorFields() {
$("#firstChoice_row").show();
$("#secondChoice_row").show();
}
function showAthleticFields() {
$("#sport_row").show();
$("#meetCoach_row").show();
}
// hide on initial load
hideEQSFields();
hideMajorFields();
hideAthleticFields();
$('[name="eqs_student"]').change( function () {
hideEQSFields();
switch($('[name="eqs_student"]').val()) {
case "Yes":
showEQSFields();
break;
case "No":
hideEQSFields();
$("#eqs_seat").empty();
$("#eqs_visit_time").empty();
break;
default:
console.log("default -- no action");
}
});
$('[name="meetProf"]').change( function () {
hideMajorFields();
switch($('[name="meetProf"]').val()) {
case "Yes":
showMajorFields();
break;
case "No":
hideMajorFields();
$("#firstChoice").empty();
$("#secondChoice").empty();
break;
default:
console.log("default -- no action");
}
});
$('[name="interested_athletics"]').change( function () {
hideAthleticFields();
switch($('[name="interested_athletics"]').val()) {
case "Yes":
showAthleticFields();
break;
case "No":
hideAthleticFields();
$("#sport").empty();
$("#meetCoach").empty();
break;
default:
console.log("default -- no action");
}
});
What this code does is hides the optional EQS fields, optional Major fields, and optional Athletics fields by default. If "Yes" is selected as the answer for their "dependent" fields, then the appropriate fields will show.
They are divided into those three categories to prevent show/hide conflicts between the different sets. For example, a student may be interested in EQS, but not in Athletics. We don't want the EQS fields to disappear if they chose "No" for athletics, so I gave each one their own "set". (I don't know if this is best practice or not, but please feel free to enlighten me either way :) )
Now to the problem...
The problem is that my code only hides the fields when the state of the determining field is changed from Yes to No. It doesn't actually clear the data from those fields.
So, what can happen is that a candidate will be filling out the form and select "Yes" for "Interested in Athletics", then select "Baseball" for the "Sport", and "Yes" for "Meet with a Coach". But then let's say that student changes their mind, and says "No" for "Interested in Athletics". The form will submit "No" for "Interested in Athletics", but it will still submit "Baseball" and "Meet with a Coach" to the database.
I'm sure the Admissions people will be able to figure it out, but the form itself will still be "broken".
I am wanting to know if it is possible to clear the values from those fields when the case is "No" for those options. Ideally, in the switch statement itself, but I'm not sure how to go about it.
I apologize again for the length of this post, though I am grateful that Treehouse doesn't set character limits! :)
Thanks!
[UPDATE:]
I have edited the code above to reflect the current "empty" states that I have set, and because I have moved the .shows into their own functions (up with the functions that have the .hides)
1 Answer
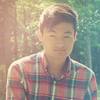
Zhihao Wang
9,687 PointsHi William,
Here's a simple solution.
function hideEQSFields() {
$("#eqs_seat_row").hide();
$("#eqs_visit_time_row").hide();
$("#eqs_seat").val(" ");
}
The trick is to use the .val() internal function of jquery to set the values of the inputs to a space, or blank.
EDIT: If the eqs_seat is the form itself, make sure you specify that you are clearing the values of the inputs in the form by declaring:
$('#eqs_seat input')
or the appropriate selector.
Cheers.

William J. Terrell
17,403 PointsThanks! eqs_seat, and many of the others, are < select > items, with < option >s that drop down, but the initial option is "& nbsp;" (some of the other initial options are "Please select a time...", so I assume I would just put that for the .val())
So, I would want to write something like this, then?
function hideEQSFields() {
$("#eqs_seat_row").hide();
$("#eqs_visit_time_row").hide();
$("#eqs_seat select option").val(" ");
$("#eqs_visit_time select option").val("Please select a time...");
}
Thanks!
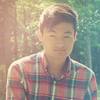
Zhihao Wang
9,687 PointsYou could definitely try that and I think it should work. However if you're looking for a more permanent and clean solution, I would use the .empty() function. The .empty() function will clear all of the values from your drop down, and it is permanent, so if the user changed their mind again and checked yes, the drop down will no longer have any options.
This is a problem, if you insert .empty() into your hide functions. However, if you were to use it on all the hidden select and drop downs when the user submitted the form, this would be the best solution to your problem. This way, instead of showing " " or "Please select a time..." in the database, it would be blank.

William J. Terrell
17,403 PointsThat seems like it works, I've also modified the code so that the code to show those rows are in their own functions as well, as opposed to in the Case "Yes":.
The only problem that I can see, though, is that, if a visitor comes onto the form and selects "No" for "Would you like to meet with a professor?", but then changes their mind and selects "Yes" instead, the fields that are dependent on that choice ("firstChoice" and "secondChoice") will be empty, and, to the visitor, the form will appear as though it is broken.
I did try the other method, using:
$("#eqs_seat select option").val(" ");
$("#eqs_visit_time select option").val("Please select a time...");
but for some reason, those fields were visible regardless of the condition...
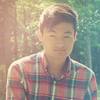
Zhihao Wang
9,687 Pointsempty() would only be practical if you execute it at the end of the form, for example, when the user pushes next or submits the form, that's when you should check for all forms that are hidden, and then run empty() to those fields.
William J. Terrell
17,403 PointsWilliam J. Terrell
17,403 PointsBy the way, if it helps, the names of the fields that I am wanting to clear are the same as the names of the rows that I am setting to .show or .hide, only without the "_row" in the name.
So, for example, for the EQS fields, I want it to either show or hide the "eqs_seat_row" and the "eqs_visit_time_row", but I want to be able to clear the "eqs_seat" and "eqs_visit_time" fields.
If that clarifies anything at all :)
Thanks!