Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial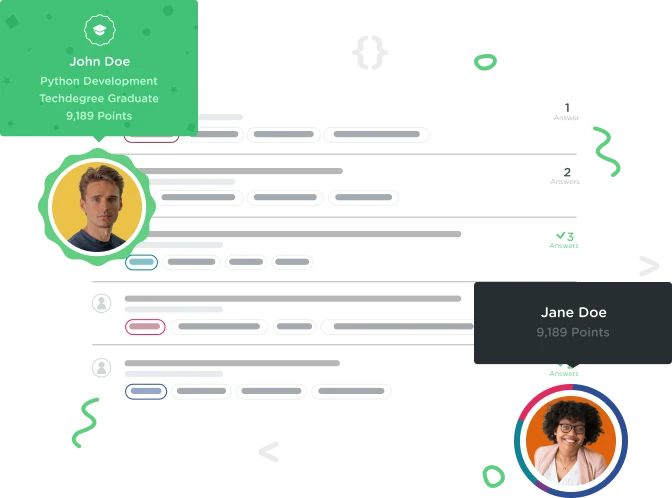

Brandy Fishback
4,223 PointsHow would I keep score in this simple quiz?
I have an array with the questions and answers. I put the answers in another array to shuffle them. Now I don't know how to access the "correctAnswer" to keep score. When I vardump it the answers come up at answer1, answer2 and answer3 instead of correctAnswer, firstIncorrectAnswer, and secondIncorrectAnswer. Here is my array and code.
<?php
include ("inc/questions.php");
session_start();
$pageTitle = "Math Quiz: Addition";
// if (!isset($_SESSION["score"])) {
// $_SESSION["score"] = 0;
//shuffle questions and hold them in $_SESSION Variable
if(!$_SESSION) {
shuffle($questions);
$_SESSION['questions'] = $questions;
}
//keep track of what question the quiz is on
if ((!isset($_SESSION["counter"]) || $_SESSION["counter"] >9)){
$_SESSION["counter"] = 1;
} else {
$_SESSION["counter"] += 1;
}
//set the counter to 0
$index = $_SESSION["counter"] - 1;
//put the answers in an array ro shuffle them
$choices = [
$_SESSION['questions'][$index]["correctAnswer"],
$_SESSION['questions'][$index]["firstIncorrectAnswer"],
$_SESSION['questions'][$index]["secondIncorrectAnswer"],
];
shuffle($choices);
?>
<html lang="en">
<head>
<meta charset="UTF-8">
<title><?php echo "$pageTitle";?> </title>
<link href='https://fonts.googleapis.com/css?family=Playfair+Display:400,400italic,700,700italic' rel='stylesheet' type='text/css'>
<link rel="stylesheet" href="css/normalize.css">
<link rel="stylesheet" href="css/styles.css">
</head>
<body>
<div class="container">
<div id="quiz-box">
<p class="breadcrumbs"> <?php echo "Question Number " . $_SESSION["counter"] . " of " . "10"; ?>
<p class="quiz"><?php echo "What is " . $_SESSION['questions'][$index]["leftAdder"] . " + " . $_SESSION['questions'][$index]["rightAdder"];?></p>
<form action="index.php" method="post">
<input type="hidden" name="id" value="0"/>
<input type="submit" class="btn" name="answer1" value="<?php echo $choices[0];?>" />
<input type="submit" class="btn" name="answer2" value="<?php echo $choices[1];?>" />
<input type="submit" class="btn" name="answer3" value="<?php echo $choices[2];?>" />
</form>
<?php
if($_SESSION['counter'] == 10) {
session_destroy();
} ?>
</div>
</div>
</body>
</html>
My Array
<?php
$questions[] =
[
"leftAdder" => 3,
"rightAdder" => 4,
"correctAnswer" => 7,
"firstIncorrectAnswer" => 8,
"secondIncorrectAnswer" => 10,
];
$questions[] =
[
"leftAdder" => 16,
"rightAdder" => 32,
"correctAnswer" => 48,
"firstIncorrectAnswer" => 52,
"secondIncorrectAnswer" => 61,
];
$questions[] =
[
"leftAdder" => 45,
"rightAdder" => 12,
"correctAnswer" => 57,
"firstIncorrectAnswer" => 63,
"secondIncorrectAnswer" => 55,
];
$questions[] =
[
"leftAdder" => 42,
"rightAdder" => 18,
"correctAnswer" => 60,
"firstIncorrectAnswer" => 69,
"secondIncorrectAnswer" => 57
];
$questions[] =
[
"leftAdder" => 96,
"rightAdder" => 20,
"correctAnswer" => 116,
"firstIncorrectAnswer" => 120,
"secondIncorrectAnswer" => 110
];
$questions[] =
[
"leftAdder" => 44,
"rightAdder" => 85,
"correctAnswer" => 129,
"firstIncorrectAnswer" => 132,
"secondIncorrectAnswer" => 126
];
$questions[] =
[
"leftAdder" => 51,
"rightAdder" => 35,
"correctAnswer" => 86,
"firstIncorrectAnswer" => 96,
"secondIncorrectAnswer" => 82
];
$questions[] =
[
"leftAdder" => 5,
"rightAdder" => 61,
"correctAnswer" => 66,
"firstIncorrectAnswer" => 65,
"secondIncorrectAnswer" => 74
];
$questions[] =
[
"leftAdder" => 26,
"rightAdder" => 19,
"correctAnswer" => 45,
"firstIncorrectAnswer" => 40,
"secondIncorrectAnswer" => 39
];
$questions[] =
[
"leftAdder" => 26,
"rightAdder" => 35,
"correctAnswer" => 61,
"firstIncorrectAnswer" => 59,
"secondIncorrectAnswer" => 51
];