Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial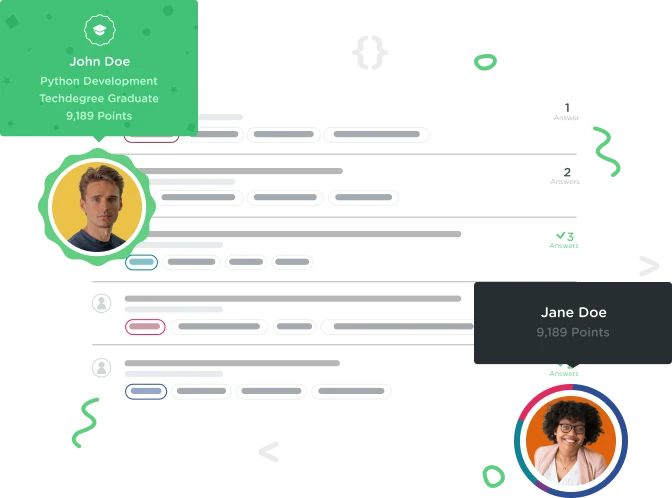
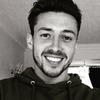
Shaun Kelly
35,560 PointsHow would i let the user change the background colour of the page from a selection of colours?
Hi guys,
I want to create something similar to this: https://www.dulux.co.uk/shop/colours/greens/
Once a colour is selected it needs to change the colour of the background.
Any help would be appreciated! Thanks in advance!
1 Answer
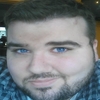
Marcus Parsons
15,719 PointsHere is a simple color changer I created for ya, Shaun Kelly. It utilizes a tiny bit of jQuery to get the process done. Each div is a block of width and height of 100 px with the class colorBlock
and once you click on a color of block, it sets the background-color
of the body
to the background-color
of the colorBlock
selected.
<!DOCTYPE html>
<html>
<head>
<title>Color Test</title>
<style>
.colorBlock {
width: 100px;
height: 100px;
}
#Black {
background-color: #000;
}
#Blue {
background-color: #00f;
}
#Red {
background-color: #f00;
}
#Green {
background-color: #0f0;
}
</style>
</head>
<body>
<div class="colorBlock" id="Black"></div>
<div class="colorBlock" id="Blue"></div>
<div class="colorBlock" id="Red"></div>
<div class="colorBlock" id="Green"></div>
<script src="http://code.jquery.com/jquery-1.11.0.min.js" type="text/javascript" charset="utf-8"></script>
<script type="text/javascript" charset="utf-8">
//Create anonymous function that listens for a click on anything with the class colorBlock
$(".colorBlock").click(function() {
//Get the background color of the clicked color block by using the this object
var $backgroundColor = $(this).css("background-color");
//Set the body's background-color to the received background-color
$("body").css("background-color", $backgroundColor);
});
</script>
</body>
</html>