Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial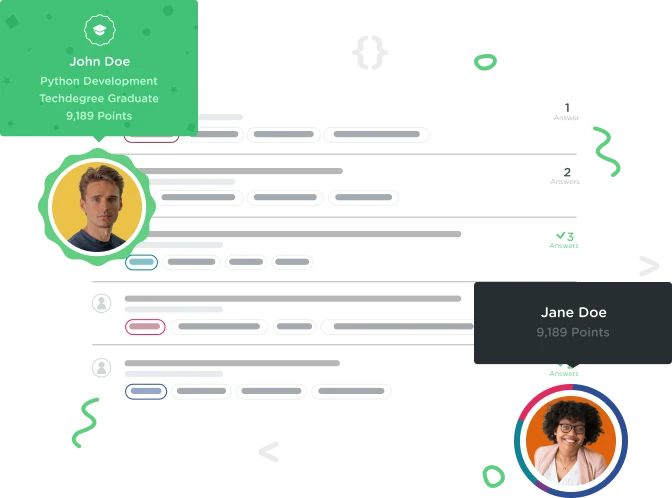
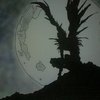
Lucas Santos
19,315 PointsHow would I loop through all of my new user instance and get their name property?
var user = {
name: 'anon',
rank: 0,
age: 0,
online: function(){
print("This user is online");
}
};
function User(name, rank, age){
this.name = name;
this.rank = rank;
this.age = age;
}
User.prototype = user;
bob = new User("Bob", 1000, 30);
roy = new User("Roy", 500, 26);
jake = new User("Jake", 300, 18);
So as you see I have 3 new user objects of
bob = new User("Bob", 1000, 30);
roy = new User("Roy", 500, 26);
jake = new User("Jake", 300, 19);
Now the question here is how can I loop through all of my user objects and get just their names or age.
I did take another javascript course on looping through objects and arrays but they did not cover looping through prototype objects like this one. What I learned on that one is looping through objects in arrays like this:
var users = [
{ name: "Bob",
rank: 1000,
age: 30
},
{ name: "Roy",
rank: 500,
age: 26
},
{ name: "Jake",
rank: 300,
age: 19
},
];
for( i = 0; i < users.length; i++ ){
document.write('<p>' + users[i].name + '</p>');
}
So this would loop through all those created objects in the array and grab the names property like:
- Bob
- Roy
- Jake
Now can someone please explain the difference on creating objects within arrays and prototyping an object. I understand that prototyping is less work but I don't understand why people would want to create objects in arrays like that instead of prototyping objects like in this course.
So if someone can please:
A. Explain how to loop through the prototypes objects and grab the name properties like I did in the users array with objects in them.
and
B. Explain how those are different meaning creating objects within arrays and prototyping objects and which one I should use more often. Just more explanation on the difference between both because it can get confusing.
1 Answer
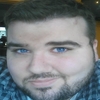
Marcus Parsons
15,719 PointsHey Lucas Santos,
A. You can't loop through those prototype objects because they are separate objects. You'd have to call each one individually.
B. I think it is MUCH easier to use an array to store data such as this, and I'll show you why. I'm going to adapt your first code for creating a new user into your 2nd code so that you can easily print off values of those objects you have in your array:
var users = [
{ name: "Bob",
rank: 1000,
age: 30
},
{ name: "Roy",
rank: 500,
age: 26
},
{ name: "Jake",
rank: 300,
age: 19
}
];
function User(name, rank, age){
//This command pushes the new data received into the users object
//The key name is always received from the originating object i.e. the 1st name
//The value is always received from the function or an outside variable i.e. the 2nd name you see
users.push({ name : name, rank : rank, age : age });
}
//Anytime you want to create a new user just call this function
User("Marcus", 15000, 27);
for( i = 0; i < users.length; i++ ){
document.write('<p>' + users[i].name + '</p>');
}
Lucas Santos
19,315 PointsLucas Santos
19,315 PointsI see very nice using the constructor to push arguments in the array with an object but let's say I use your method and I have 1000 users how would I get a certain 1 .. like theirs 1000 users and I want to grab the Jake object, how would I be able to selects that certain one?
Also then what is the benefits or the point of making an object with prototype?
Marcus Parsons
15,719 PointsMarcus Parsons
15,719 PointsPart A
Easy peezy, lemon squeezy. :)
Let's say you were looking for "Jake" in your array. You create this "search" object as part of a function called "lookup" that holds the value of the name of the property you are looking for. In this case, we want to look up someone by their "name" with a given value, "Jake". We specify the value when we call the function and we get:
Part B
I haven't personally found any use for prototyping objects, but I'm sure that someone else has actually used them in a project and would like to share their experiences.
Lucas Santos
19,315 PointsLucas Santos
19,315 PointsI see thats a good way to go about it, thanks a ton Marcus. One problem that I really have is "How" to get certain things done or accomplished in javascript. I mean I know the concept of javascript and have a really good understanding but when it comes to "how" to do certain things thats when I get stuck.
Marcus Parsons
15,719 PointsMarcus Parsons
15,719 PointsAnytime you have questions, don't be afraid to ask! Happy Coding, Lucas!