Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial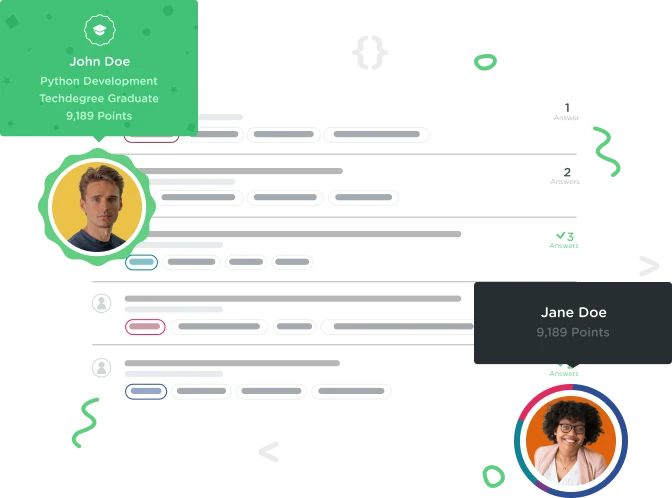

Ryan Lail
4,386 PointsHow would I make my code carry on if the user enters anything different to an int?
Well I was thinking about making my code carry on and continue asking the user for inputs instead of completely ending the game. Heres my code, is this possible?
import random
number = random.randint(1,10)
correct = False
count = 0
limit = 5
while correct == False:
count += 1
user_input = (input("Pick a number between 1 and 10: "))
try:
user_input = int(user_input)
except:
print("That's not a whole number!")
break
if user_input == number:
print("Congratz. You got it in {} tries".format(count))
correct = True
elif user_input < number:
print("Too low")
elif user_input > number:
print("Too high")
else:
print("Invalid number")
1 Answer

Charles Lee
17,825 PointsWhat you're looking for is the continue
keyword instead of break
.
import random
number = random.randint(1,10)
correct = False
count = 0
limit = 5
while not correct:
count += 1
user_input = (input("Pick a number between 1 and 10: "))
try:
user_input = int(user_input)
except ValueError:
print("That's not a whole number!")
continue # Restarts the outer loop.
if user_input == number:
return "Congratz. You got it in {} tries".format(count)
elif user_input < number:
print("Too low")
elif user_input > number:
print("Too high")
else:
print("Invalid number")
Few changes beyond your question that might be helpful:
- Change the condition in the while loop to something more readable.
- Explicitly state the exception.
- Simply return when the user correctly guesses the number.
Hope this helps. :]
Ryan Lail
4,386 PointsRyan Lail
4,386 PointsThanks, really helps. Just a few questions though: 1 What does 'Explicitly state the exception' mean? 2 Why is it better to return rather than print once the user correctly guesses the number? 3 What does 'except ValueError' mean? (I assume it has something to do with my first question)
Charles Lee
17,825 PointsCharles Lee
17,825 PointsWhen you use the except statement, it's better practice to state which exceptions you want to catch. By having a 'bare' except, you're potentially letting any exception be caught through it instead of specific ones. Here's a more detailed SO post about explicitly stating exceptions: http://stackoverflow.com/questions/14797375/should-i-always-specify-an-exception-type-in-except-statements
It's not necessarily better, but imagine having this as one method. You want to return from the method instead of printing. However, in this case, you're definitely right for going for the print statement. I think it gets to a point there you start designing everything as a method and calling that method after.
Yes it does. You can read more about the different types of exceptions in Python's docs: https://docs.python.org/3.5/library/exceptions.html#ValueError.
Ryan Lail
4,386 PointsRyan Lail
4,386 PointsPerfect! Thanks. Here's my code now, I think you made it a LOT better!!! :)
Charles Lee
17,825 PointsCharles Lee
17,825 PointsLooks pretty good! Small change.
You also want to be careful of floats. int(5.5) still works, but 5.5 wouldn't be a valid whole number.