Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial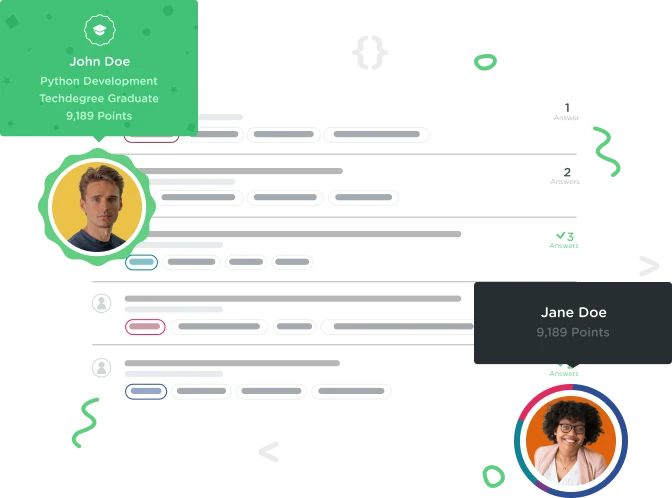
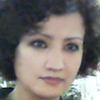
Durdona Abdusamikovna
1,553 PointsHow would I separate array's elements if it has more than two values ?
Hello there,
My question is related to Two-Dimensional Array . I have arrays with more than two values and without specifying each array's location I am iterating over arrays with arrays[k] and I still see all elements in the array however, if I use arrays[k][0] + arrays[k][1] + arrays[k][2] I don't see a comma between each element. Please bear with me my question seems long this time :) ... I have two questions based on that
If I don't specify each element's location and iterate with simply arrays[k] I need a space between each element where in my code I can add it? Is it still acceptable to use just array[k] since I see all three elements of the array?
If my way is not right and I need to use arrays[k][0] + arrays[k][1] + arrays[k][2] How can I separate each element with comma because when I run this way no comma appears just a space .
Thank you beforehand
// creating Two-Dimentional Arrays
var list = [ // outter array
['I am first 0 index ', '0 index\'s first element ', 'Testing adding the second element'],
['I am second 1st index ', '1st index\'s first element ', 'Testing adding the second element'],
['I am third 2nd index ', '2nd index\'s first element ', 'Testing adding the second element'],
['I am fourth 3rd index ', '3rd index\'s first element ', 'Testing adding the second element'],
['I am fifth 4th index ', '4th index\'s first element ', 'Testing adding the second element']
];
document.write('<h1>Here is an example of Two-Dimentional Array </h1>');
function print (message){
document.write(message);
}
function printArrays (arrays){
var listHTML = '<ol>';
for(var k = 0; k < arrays.length; k += 1){
//or listHTML +='<li>' + arrays[k][0] + arrays[k][1] + arrays[k][2] +'</li>';
listHTML += "<li>" + arrays[k] + "</li>";
}
listHTML += '</ol>';
print(listHTML);
}
printArrays(list);
P.S. I tried to apply .join() method and I believe it should work with more than two elements
4 Answers
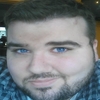
Marcus Parsons
15,719 PointsHiya Durdona!
Good to see you again! The difference between the two methods is how each piece of data is interpreted by the JavaScript parser.
Let's look at the lines that are causing differences:
listHTML += "<li>" + arrays[k] + "</li>";
What the above line is doing is getting all of the values of each index of the array, which is still an array, because it's an array within an array. And because it's an array, JavaScript does type conversion on the array and translates it into a string which always has commas separating each individual index's value.
This line:
listHTML +='<li>' + arrays[k][0] + arrays[k][1] + arrays[k][2] +'</li>';
It gets the specific value of each index which happens to be a string. Since these are already strings, no type conversion needs to be done and so it just concatenates the actual string to the other portions of the string. When you are referencing specific indices that are strings, you'll have to manually put in the comma (or other separator) yourself:
listHTML +='<li>' + arrays[k][0] + "," + arrays[k][1] + "," + arrays[k][2] +'</li>';
Now you will see the same output with both commands.

Jason Anello
Courses Plus Student 94,610 PointsHi Durdona,
You didn't give an example of how you tried to use the .join()
method so I'm not sure why it didn't work out for you.
This problem is a good use case for the join method and probably the easiest way to solve the problem.
Concatenation of the individual array elements is going to be unwieldy for arrays with more than a few values.
From what I can gather you wanted to separate each array element with a comma and then a space.
Did you try:
listHTML += "<li>" + arrays[k].join(', ') + "</li>";
This worked for me when testing in the console. You can change the separator to whatever you like.
Also, I would recommend not adding trailing spaces to the 1st and 2nd elements of your inner arrays. This gives you less flexibility in controlling the output.
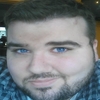
Marcus Parsons
15,719 PointsYou're right. I pretty much re-wrote the join() method lol She was using for loops so I figured I'd keep the pattern going and explain how it can be done with logic, instead of just methods, although join() is easier than the for-loop method.
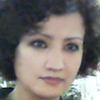
Durdona Abdusamikovna
1,553 PointsThank you I will examine it shortly :)
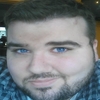
Marcus Parsons
15,719 PointsYou're welcome =]
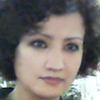
Durdona Abdusamikovna
1,553 PointsI appreciate your answer Jason. I just tried it works fine
I didn't try to append .join() method to the array itself now I know that it is doable :)
Thank you!
Marcus Parsons
15,719 PointsMarcus Parsons
15,719 PointsThe difference between using
arrays[k]
and using specificarrays[k][#]
is that you can specify another separator while it's being initialized. Let's say we wanted semicolons instead of commas in between each value:listHTML +='<li>' + arrays[k][0] + ";" + arrays[k][1] + ";" + arrays[k][2] +'</li>';
Also, you would want to do a second for loop to iterate over each secondary index in the array, as well, instead of that static piece of code so that you could have as many values as you wish within any portion of your array.
Durdona Abdusamikovna
1,553 PointsDurdona Abdusamikovna
1,553 PointsI could have just used + ", " + between them wow it is so simply and didn't cross my mind. I was trying to apply a hard way as usual :) with .join() method. Gotta try to make it simple :)
Marcus Parsons
15,719 PointsMarcus Parsons
15,719 PointsAbsolutely! Here's a more modular version of your full code. This one will iterate over all values contained in your arrays so that you can add as my indices as you wish. Take a while to examine it and see exactly what it's doing: