Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial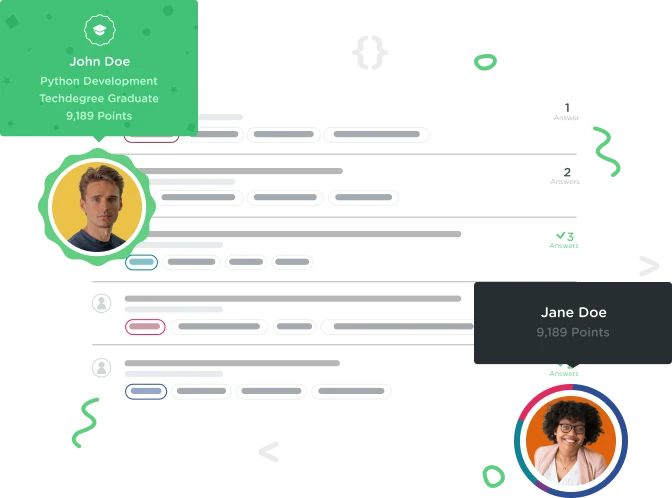
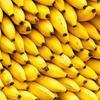
David Accomazzo
15,986 PointsHow would I throw a user-friendly error for the number of tickets not being a string?
Code looks like this so far:
try:
num_tickets = input("How many tickets would you like, {}? ".format(name))
num_tickets = int(num_tickets)
# Raise a ValueError if the request is for more tickets than are available
if num_tickets > tickets_remaining:
raise ValueError('There are only {} tickets remaining.'.format(tickets_remaining))
except ValueError as err:
# include the error text in the output
print("Oh no, we ran into an issue. {}. Please try again.".format(err))
How do we throw an error if int(num_tickets) throws a ValueError? I'm not sure what the syntax for that looks like in Python. Currently it just throws the generic error message if num_tickets is a string. I would like to have it throw something like "You must enter a number, not letters, here!"
2 Answers
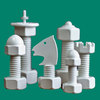
Steven Parker
230,274 PointsOne way would be to peek at the contents of the message that would be issued, and replace it with a custom message instead:
except ValueError as err:
if ("invalid literal" in str(err)):
# use custom message for bad characters
print("You must enter a number, not letters, here!")
else:
# include the error text in the output
print("Oh no, we ran into an issue. {} Please try again.".format(err))
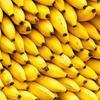
David Accomazzo
15,986 PointsSounds good to me! Thanks