Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial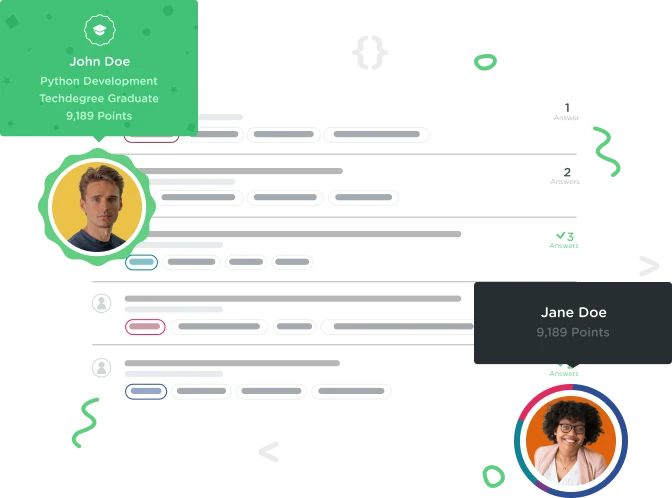
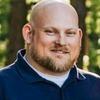
Brandon Canter
8,964 PointsHow would one go about saving selected (options) in select boxes when form page is reloaded?
For example: (This does not work.)
select name="hearAbout" value="PHP CODE HERE">
option value="Google">Google Search</option>
option value="Referral">Referral</option>
option value="Direct-Mail">Direct Mail</option>
/select>
Id like to be able to retain this information as well as information placed into standard (input type="text") boxes.
Any help is appreciated.
3 Answers
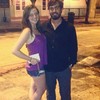
Kyle Gunby
23,838 PointsPHP has global array variables that will store this information based on the name attribute in your input tag. How you would get them depends on the method in your form tag.
example. <input type="text" name="example1"> To get this value, it's stored in a global array. Which one depends on your forms method. the two options would be: $_POST['example1']; and $_GET['example1'];
It's the same principle with select statements. You would use the name attribute declared in the select statement. <select name ="example2"> option value="Google">Google Search</option>
option value="Referral">Referral</option>
option value="Direct-Mail">Direct Mail</option>
</select>
To get the value, you would use one of the array variables. $_POST['example2']; and $_GET['example2'];
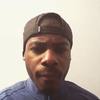
Tunde Adegoroye
20,597 Pointsyou'd get name value of the select and get the value from the post and into a variable and then echo the value from the variable into the value of the select.
// At the top of your php file
$about = $_POST["hearAbout"];
//In the value of the select option
// Check if the variable has a value
if(isset($about)){
// If it does echo out the value but remove any special characters or unwanted characters
echo htmlspecialchars($about);
}
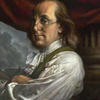
Jeff Busch
19,287 PointsHi Brandon,
I've been playing around with $_POST a little and it seems that post doesn't retain the values from page to page. To accomplish this I used the $_SESSION variable. To use it you need to start a session on every page. I'll provide some code below to illustrate. Hope this is what you had in mind.
Jeff
<php session_start(); ?>
<form action="process.php" method="post">
<h1>Shirt Order Form</h1>
<label for="color">Shirt Color</label>
<select id="color" name="shirt_color">
<option value="red">Red</option>
<option value="yellow">Yellow</option>
<option value="purple">Purple</option>
<option value="blue">Blue</option>
<option value="green">Green</option>
<option value="orange">Orange</option>
</select>
<input type="submit" value="Submit">
</form>
The print_r(); lines are just a more readable var_dump().
process.php:
<?php
session_start();
$shirtColor = $_POST['shirt_color'];
$_SESSION["shirtColor2"] = $shirtColor;
$shirtColor2 = $_SESSION['shirtColor2'];
echo $shirtColor . "<hr>";
echo $shirtColor2 . "<hr>";
echo "<pre><p>SESSION</p>";
print_r($_SESSION);
echo "</pre><hr>";
echo "<pre><p>POST</p>";
print_r($_POST);
echo "</pre>";
?>
<a href="someOtherFile.php">Some Other File</a>
someOtherFile.php
<?php
session_start();
$shirtColor = $_POST['shirt_color'];
$shirtColor2 = $_SESSION["shirtColor2"];
echo $shirtColor . "<hr>";
echo $shirtColor2;
echo "<pre><p>SESSION</p>";
print_r($_SESSION);
echo "</pre><hr>";
echo "<pre><p>POST</p>";
print_r($_POST);
echo "</pre>";
session_destroy();
?>