Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial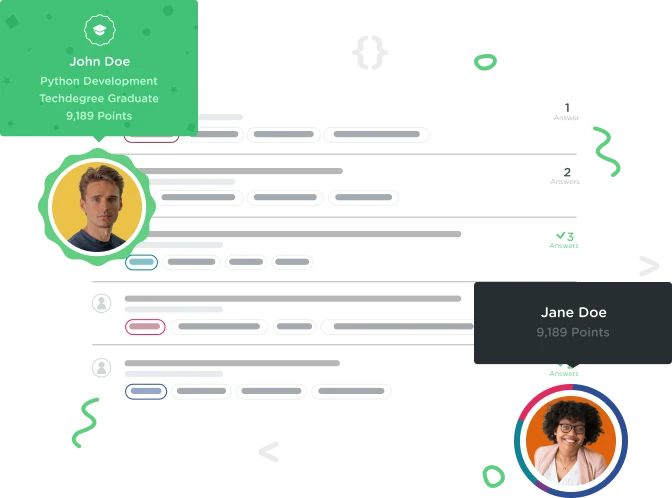
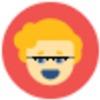
Tom Stanley
15,308 PointsHow would you destructure props in AddPlayerForm.js?
If I try to destructure this.props.addPlayer
in the render method of AddPlayerForm, I get this error:
./src/components/AddPlayerForm.js
Line 16: 'addPlayer' is not defined no-undef
Is there a way around this, or can't you destructure this function from props in this case?
import React, { Component } from "react";
class AddPlayerForm extends Component {
state = {
value: ""
};
handleValueChange = (event) => {
this.setState({ value: event.target.value })
}
handleSubmit = (e) => {
e.preventDefault();
addPlayer(this.state.value); // addPlayer this isn't defined yet
this.setState({
value: ""
})
}
render() {
const { addPlayer } = this.props;
return (
<form onSubmit={this.handleSubmit}>
<input
type="text"
placeholder="Enter a player's name"
value={ this.state.value }
onChange={this.handleValueChange}
/>
<input
type="submit"
value="Add Player"
/>
</form>
);
}
}
export default AddPlayerForm;
3 Answers

Antoine Venco
4,787 PointsYou need to declare the variable inside of your function in order for it to access the value, not sure if this is correct but it's working :
handleSubmit = (e) => {
const { addPlayer } = this.props;
e.preventDefault();
addPlayer(this.state.value); // addPlayer this isn't defined yet
this.setState({
value: ""
})
}
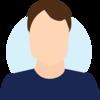
Mike Hatch
14,940 PointsHi Antoine,
It may work, but is that actually considered "Destructuring"? It doesn't seem to take away anything, it only adds. Also, addPlayer
has already been declared in App.js. Take a look at the very bottom of the file:
<AddPlayerForm addPlayer={this.handleAddPlayer} />
As I understand it, addPlayer
is a prop and is assigned the function handleAddPlayer
. (Just a fellow student still learning, so I'm not positive I used correct terminology or not.)

Charlie Prator
Front End Web Development Techdegree Graduate 19,854 PointsWhat about writing the destructured variable assignment from within the handleSubmit() method?
import React, { Component } from 'react';
class AddPlayerForm extends Component {
// const { addPlayer } = this.props;
state = {
value: ''
};
handleValueChange = (e) => {
this.setState({ value: e.target.value });
}
handleSubmit = (e) => {
const { addPlayer } = this.props; // destructured variable assignment
e.preventDefault();
addPlayer(this.state.value);
this.setState({value: ''});
}
render() {
// Where I originally put the destructure variable assignment
return (
<form onSubmit={this.handleSubmit}>
<input type="text" value={this.state.value} onChange={this.handleValueChange} placeholder="Enter a player's name"/>
<input type="submit" value="Add Player"/>
</form>
);
}
}
export default AddPlayerForm;

Nick Huemmer
Front End Web Development Techdegree Graduate 26,840 PointsThanks - I implemented this solution and it worked!
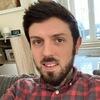
Joe Mottershaw
5,866 PointsI agree with Mike Hatch, I think that's why Guil said he's going to stop there cause there isn't actually any gain from doing it in the AddPlayerForm
component as the render method references state (which you don't destructure afaik) or it's own methods (handleSubmit
and handleValueChange
). The only place this.props
is used is in the handleSubmit
method and since you're only referencing a single prop, adding a whole line for destructuring props isn't really required, if there were more props used I could see the benefit, same goes within the render
method.
I guess it also depends on what you (and/or your team) decide on in terms of being consistent, you might decide that even if just one prop is used/available, you destructure regardless. One it might increase the readability of the codebase and second if it's expanded upon at a later date the process has already been started. I'd say just be consistent in whatever choice you make.
Mike Hatch
14,940 PointsMike Hatch
14,940 PointsGood question. I'm currently under the belief that you can't. There are no props to destructure here. There's only state.