Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial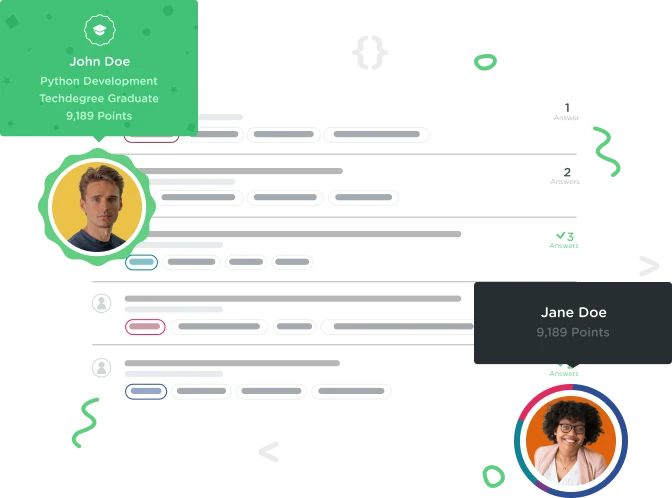

Erik Lopez
415 PointsHow would you do a nested for loop if you wanted to randomize the combinations between each of the two arrays?
For instance, if you didn't want a list of all the combinations starting with caramel, then wanted each of the combinations with cinnamon, then watermelon, et al. Instead, you wanted a randomized version where any one of the four sweets started and any one of the four savory was appended to a sweet. What would that look like in code?
Marek Mikos
314 PointsMarek Mikos
314 PointsHi,
I'm new to java so my solution might not be the best, but I have come up with following solution. I have converted array to list and the used shuffle from Collection: (example with odd and even numbers)
import java.io.Console; import java.util.*; public class nestedForLoops {
}