Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial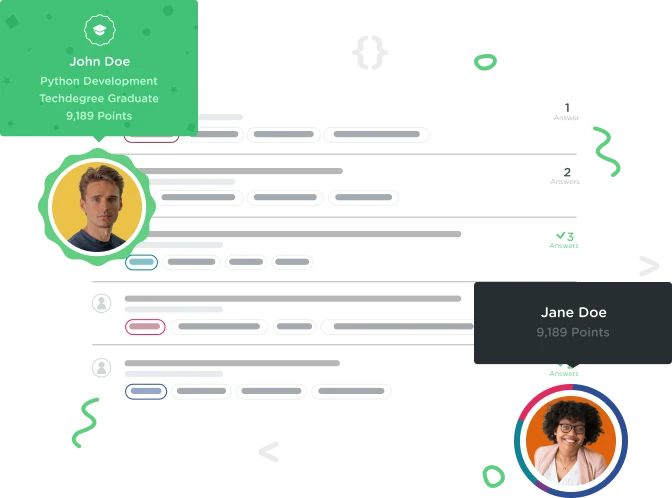

Max Gerek
2,718 PointsHow would you edit the code to account for the browser update to print each time?
How do you account for the important update?
1 Answer
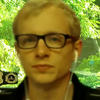
Bryan Land
10,306 PointsThis all stems around the fact that the browser only renders document.write methods in the DOM after it exits its JavaScript or rendering mode. The only solution is to use a different method of getting the search item to using a button with an event listener. See this example:
HTML:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Search the Store</title>
<link rel="stylesheet" href="css/styles.css">
</head>
<body>
<h1>Search the Store</h1>
<button>Search</button>
<p id="output"></p>
<script src="js/elements.js"></script>
</body>
</html>
JavaScript:
// button that allows for search
document.querySelector('button').addEventListener('click', function() {
// first it opens a prompt to type an item and store it in a variable
search = prompt("Search for a product in our store. Type 'list' to show all of the produce in stock and 'quit' to exit.")
// sets a variable to print out in the paragraph element with id output
var outItem = document.getElementById('output')
// checks if the item is in the array and prints different for each
// this overwrites what was last searched, so you could add more loop
// logic to make it print every search if you want...
if (inStock.includes(search)) {
outItem.innerHTML = "We do have " + search + ".";
} else {
outItem.innerHTML = "Sorry, we don't have " + search + ". Try going to Costco...";
}
})
Keep in mind though that this completely defeats the purpose of what we are learning here, which is how to iterate through arrays and find the items inside... Still a nice little workaround for you. If someone can refactor it to print every searched item that would be awesome!!