Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial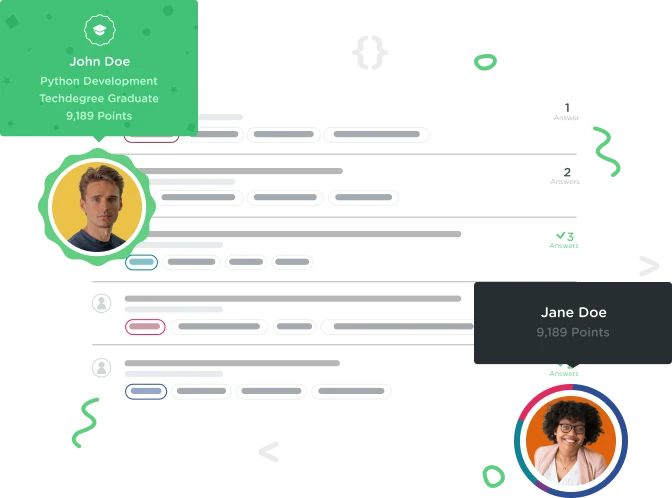
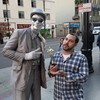
Humberto Zayas
7,405 PointsHow would you go about making this quiz with both strings and numbers or even boolean values as answers to questions?
I was about to ask -- Why did Dave use parseInt to convert the answer to a number? Isn't using the strictly equals operator enough (EX: response === answer). I realized since his example uses numbers as answers ONLY and the prompt method returns strings, he would have to turn the responses to integers.
But how would you go about checking a quiz that had both strings and numbers as answers to questions? Or even true false?
EX: quiz = [ ['number of states?', 50], ['what color is an apple', 'red'] ['True or false: The sky is red', false] ];
I was excited to correctly make the quiz program but I hadn't even thought about answers being numbers true or false, strings, numbers etc...
In his response video, he even starts with the line "lets make all our answers numbers to make it easy".
Here is my code using strings as answers only. I use strictly equals operator to check the string and response.
var correct = 0; var incorrect = 0; var question; var results; var quiz = [ ['first', 'one'], ['second', 'two'], ['third', 'three'], ];
function print(message) { document.write(message); }
for(var i = 0; i < quiz.length; i++) { question = quiz[i][0]; results = prompt(question); // I should have named my variables better...It can be confusing that results opens the prompt and is aslo overwritten below.
if (results === quiz[i][1]) { correct += 1; } else { incorrect += 1; } }
var results = 'You got ' + correct + ' correct and ' + incorrect + ' wrong.';
print(results);
1 Answer
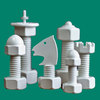
Steven Parker
229,785 PointsUsing strings directly does expand your answer possibilities, but you introduce case sensitivity. You might also want to add tolerance for leading and/or trailing white space. You can accomplish both this way:
results = prompt(question).toLowerCase().trim();
Then, store all answers as lower case.
Also, you don't need that second results variable, just do this:
print('You got ' + correct + ' correct and ' + incorrect + ' wrong.');