Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial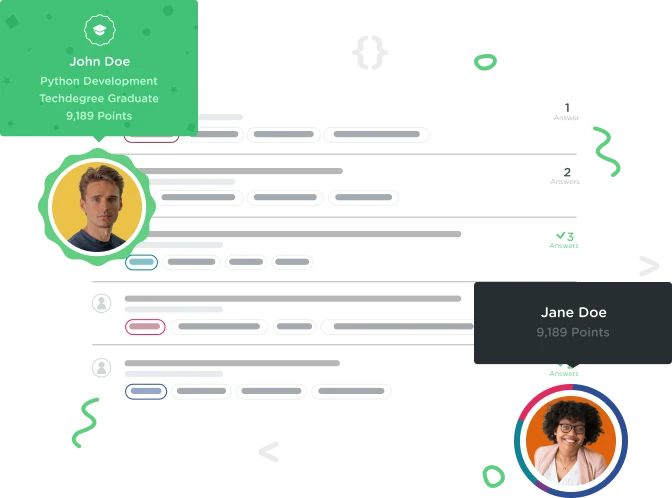
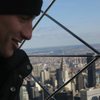
Alberto Samaniego
937 PointsHow would you print out in the console a shadow variable and a global variable from inside the function?
Just wondering if you happen to have two variables with the same name; one global and the other is a shadow of that global inside a function and you would want to use both from inside the function how would you do that?
1 Answer

Chris Shaw
26,676 PointsHi Alberto,
It really comes down to how the first variable was scoped as generally if you want an external variable to be accessible on a global level you would pass it outside of any function that would impede it from been defined on the window
object, take the following for example.
var a = 'Hello';
function b() {
var a = 'World';
console.log(window.a, a);
}
b();
This would work fine as the variable a
has been defined on the window object since it's executing in a scope that isn't controlled by anything other than window
itself, however take the following example.
;(function() {
var a = 'Hello';
function b() {
var a = 'World';
console.log(window.a, a); // undefined World
}
b();
})();
This would return undefined
for window.a
as the variable is no longer scoped to the window object which can be confusing, depending on the use cases you need this for it's much better to separate your code using a closure (i.e. the second example) which you won't have learned about yet but should after the beginners course as it helps to namespace your code away from 3rd party code which eliminates conflicts and allows for much easier access to global variables.
Alberto Samaniego
937 PointsAlberto Samaniego
937 PointsThanks Chris, exactly what I was looking for. Make sense that global variables are within the window object's scope, I just did not realize that the window object was in play... still a beginner.