Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial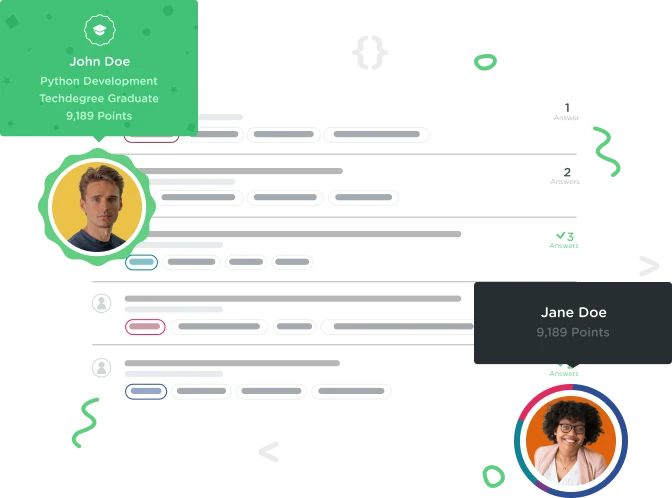

Sarai Vasquez Mariñez
3,891 PointsHow would you restructure state for AddPlayerForm.js?
Since state is an object, I figured you could also destructure that as well with const {value} = this.state;
.
Where's the best place to place that line to only have to place it once so that there's no repetitive code?
Things I have tried:
When I try to restructure it above the handleValueChange
method, it gives me an error and says there's an unexpected token.
When I leave it inside of the handleSubmit
method, it compiles as it's accessed there but at the same time, it needs to be accessed by the render method and shows up as an error in the browser.
Placing it inside the render - and only the render - method gives me a VALUE IS UNDEFINED error. Declaring the aforementioned destructured state twice - once in handleSubmit
and again in the render function makes the code compilable but this also feels like it defeats the purpose.
This is my AddPlayerForm.js
import React, { Component } from "react";
class AddPlayerForm extends Component {
/* This is where state originally is:
state = {
value: ''
}
*/
handleValueChange = (e) => {
this.setState({ value: e.target.value });
}
handleSubmit = (e) => {
const {addPlayer} = this.props;
const {value} = this.state;
e.preventDefault();
addPlayer(value);
this.setState({
value: ''
});
}
render() {
return (
<form
onSubmit={this.handleSubmit}>
<input
type="text" placeholder="Enter a player's name"
value={this.state.value}
onChange={this.handleValueChange}
/>
<input
type="submit"
value="Add Player"
/>
</form>
)
}
}
export default AddPlayerForm;