Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial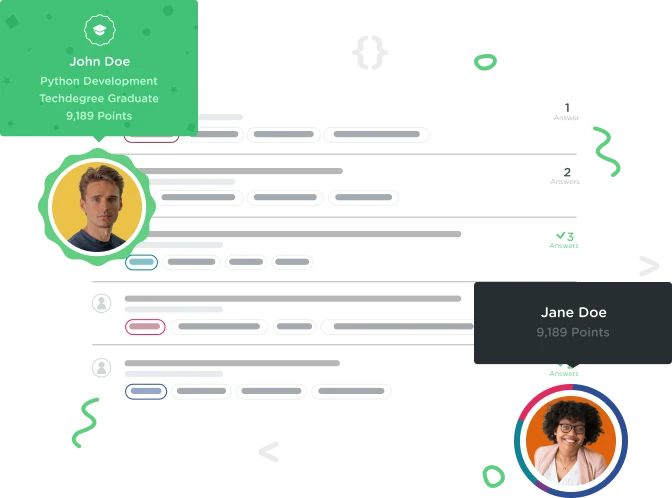

Jonathon Irizarry
9,154 PointsHow would you solve the problem at the end of this video without using bubbling?
In the previous videos, we created a new list item with the use of the createElement method, and it is a dynamically created element that is only created after the clickEventHandler is triggered. So my question is how do you even add a dynamically created element to another eventHandler to solve the problem remaining at the end of this video? Is it just as simple of a solution as bubbling?
3 Answers
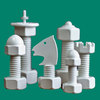
Steven Parker
230,274 PointsA big value of a delegated handler is that it takes care of elements created later.
The alternative would be to add a handler to each element as it is created. This takes a bit more code but it also uses more memory as each element has a separate handler.

Jonathon Irizarry
9,154 PointsYea it worked, but the code for some reason feels very compact almost like I'm trying to shove ten people into a small car . . . is this the only way to add a handler to the dynamically created elements without relying on delegated handlers?
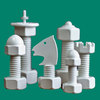
Steven Parker
230,274 PointsIn programming "compact" is good, as long as the intention is clear upon reading as it is here. Good job.
But I'm still not sure why you want to avoid "relying on delegated handlers", since that would certainly be a good approach for this situation (it might be a "best practice" and it would certainly be my preference).

Jonathon Irizarry
9,154 PointsI guess you could say it was curiosity, for some reason I like to learn the origins of everything, and I love learning about random facts in computer science even if they hold little to no value or have been deprecated.I always see you answering everyone's question with really great information. Thanks for the help!
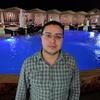
MoatazBellah Ghobashy
9,518 Pointswe can also build a function that contain the mouseover and mouseout events and call it in addItemButton.addEventListener
like this :
function hoverOver() { for (let i = 0; i < listItem.length; i++) { listItem[i].addEventListener('mouseover', () => { listItem[i].textContent = listItem[i].textContent.toUpperCase(); }); listItem[i].addEventListener('mouseout', () => { listItem[i].textContent = listItem[i].textContent.toLowerCase(); }); } }
hoverOver();
addItemButton.addEventListener('click', () => { let ul = document.getElementsByTagName('ul')[0]; let li = document.createElement('li'); li.textContent = addItemInput.value; ul.appendChild(li); addItemInput.value = ''; listItem = document.querySelectorAll('li'); hoverOver(); });
Jonathon Irizarry
9,154 PointsJonathon Irizarry
9,154 PointsSo I would need to place another callback function inside of the same callback function that is creating those new elements? Is this the correct way to add it to my code:
Steven Parker
230,274 PointsSteven Parker
230,274 PointsWithout seeing in context, this does look like it would work and attach two listeners to each new element created.
Did you test it?