Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial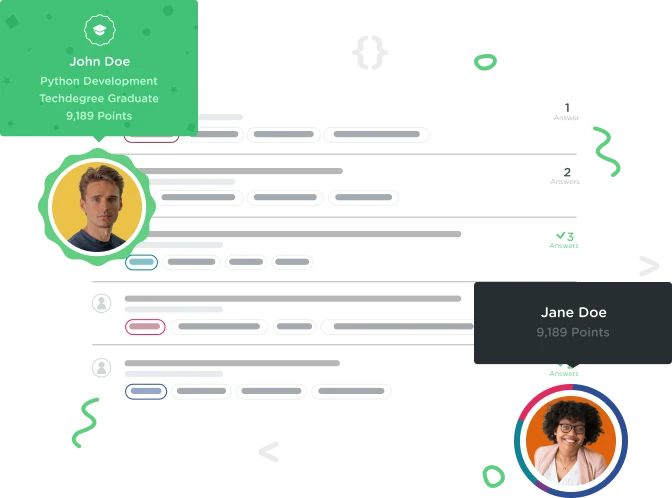

Tom Lawrence
8,685 PointsHows this for a first loop?
Hi,
Just starting to learn Javascript (very new), watched the video on basic javascript and wanted to try to get the hang of loops.
I came up with this and wondered if there was a better way to write this code? (be good to get some tips while i practice)
var counter
var loops
var remaining
counter = 0
remaining = 10
loops = 0
for (counter = counter; counter <=10 ; counter = counter +1) {
console.log("This is loop Number " + counter);
console.log("Another " + remaining + " to go" + " and I have looped " + loops + " times now!");
loops = loops +1;
remaining = 10 - loops;
}
3 Answers
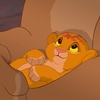
Laura Cressman
12,548 PointsHi Tom, how does this look?
Since counter will always equal loops, it is easier to just use one of the two variables. Also, counter++ is short hand for counter = counter + 1. Lastly, you can calculate remaining in the log statement without bothering with the remaining variable. What do you think?
for (var counter = 0; counter <= 10; counter++) {
console.log("This is loop #" + counter + "!");
console.log("Another " + (10 - counter) + " to go and I have looped " + counter + " times!");
}

Tom Lawrence
8,685 PointsThats great! much better mine, thanks for that.
I didn't realise you could initialise a variable within the for statement so that's handy and so was the ++ tip.
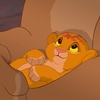
Laura Cressman
12,548 PointsYep, since the variable is only needed for the purposes of the loop, you can put it there. Also, the ++ tip only works for incrementing by 1, so if you were to increment by any other number, try counter += 2 (short hand for counter = counter + 2). You can also use that with other operations, like *=, -=, and /=. Happy coding!
James Barnett
39,199 PointsJames Barnett
39,199 PointsA clearer way to write this is...
Since JavaScript doesn't have block scope, a variable declared inside of a loop is available to the function it's inside even after the loop has been terminated.
Dino PaΕ‘kvan
Courses Plus Student 44,108 PointsDino PaΕ‘kvan
Courses Plus Student 44,108 PointsSince JavaScript doesn't have block scope...
JavaScript does have block scope. It was available through hacks (like the try/catch one), but more recently, as more and more ES6 features appear in the browsers, you can use the keyword
let
to declare block scope variables.In fact, you could write ES6 and have Traceur compile it to ES5 to achieve backwards compatibility. I believe it uses the try/catch hack for
let
.