Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial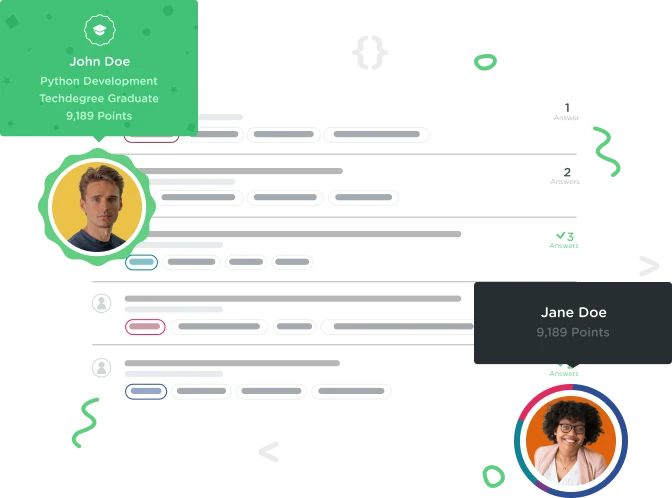

Robert Young
11,742 PointsHTML appearance errors
Hey Hampton (and the team), this is a great tutorial. I have worked my way through it, and it seems to be partially functioning, but my HTML seems to be appearing slightly incorrectly, for some unknown reason that I cannot quite figure out.
On my /todos/create screen, instead of the normal form and submit button showing, I see this code:
"<form method="POST" action="http://laravel.dev:8000/todos" accept-charset="UTF-8"><input name="_token type="hidden" value="RL2GywwhIraSrSUl760sGaYILDWzQBm948Ql0J3H"> <label for="title">List Title</label> <input name="title" type="text" id="title"> <input class="button" type="submit" value="submit"> </form> "
I am not sure quite what is happening? Instead of the blade syntax correctly displaying the form, it seems to be echoing out the html verbatim.
Here is my create.blade.php file:
@extends('layouts.main')
@section('content')
{{ Form::open( array('route' => 'todos.store') ) }}
{{ Form::label('title', 'List Title') }}
{{ Form::text('title') }}
{{ $errors->first('title', '<small class="error">:message</small>') }}
{{ Form::submit('submit', array('class' => 'button')) }}
{{ Form::close() }}
@stop
And here is my TodoListController.php file:
<?php
namespace App\Http\Controllers;
use App\Http\Requests;
use App\Http\Controllers\Controller;
use Illuminate\Support\Facades\Redirect;
use Illuminate\Http\Request;
use View;
use App\Model;
use App\User;
use App\TodoList;
use App\Http\Controllers\Validator;
use Input;
class TodoListController extends Controller { // extends the controller from Laravel
/**
* Display a listing of the resource.
*
* @return Response
*Will list out all of our ToDo lists
*/
public function __construct()
{
$this->beforeFilter('csrf', array('on' => 'post')); // puts a filter on current object but only on post methods.
}
public function index()
{
$todo_lists = \App\TodoList::all();
return View::make('todos.index')->with('todo_lists', $todo_lists);
}
/**
* Show the form for creating a new resource.
*
* @return Response
*/
public function create()
{
return View::make('todos.create');
}
/**
* Store a newly created resource in a database.
*
* @return Response
*/
public function store()
{
// define rules
$rules = array(
'title' => array('required', 'unique:todo_lists,name')
);
// pass input to validator
$validator = \Validator::make(Input::all(), $rules);
// test if input fails
if ($validator->fails()) {
return Redirect::route('todos.create')->withErrors($validator)->withInput();
}
$name = Input::get('title');
$list = new TodoList();
$list->name = $name;
$list->save();
return Redirect::route('todos.index');
}
/**
* Display the specified resource.
*
* @param int $id
* @return Response
*/
public function show($id)
{
$list = \App\TodoList::findOrFail($id);
return View::make('todos.show')->withList($list);
}
/**
* Show the form for editing the specified resource.
*
* @param int $id
* @return Response
*/
public function edit($id)
{
//
}
/**
* Update the specified resource in storage.
*
* @param int $id
* @return Response
*/
public function update($id)
{
// Updates the specified resource in the database.
}
/**
* Remove the specified resource from storage.
*
* @param int $id
* @return Response
*/
public function destroy($id)
{
// destroys the resource in the database.
}
}"
I would be very grateful for any heads up or advice on the possible issues that I may be encountering.
Thanks,
Robert London, UK
2 Answers

Greg Kaleka
39,021 PointsHi Robert,
Looks like you're using Laravel 5. This blade syntax issue is one of the differences.
This is a simple fix: in Laravel 5 Blade syntax, the two curly braces {{ }} will escape any html tags and display them to the screen verbatim. This is very helpful when displaying text that was generated by a user, as it prevents hackers from modifying your site or using SQL injection.
However, when you are simply outputting your own code, you should use one curly brace and two exclamation points: {!! !!}. The exclamation points are meant as a sort of warning not to use them when user input will be displayed.
Let me know if that fixes your issue!

mairakotsovoulou
3,907 Points{!! fixed the escaping in HTML, but I the error class is missing from foundation 6... so I changed the line {{ $errors->first('title', '<small class="error">:message</small>') }} with {!! $errors->first('title', '<span class="label alert">:message</span>') !!} and appearance is also fixed.
Joshua Campbell
8,673 PointsJoshua Campbell
8,673 PointsThis fixed the issue for me. Thank you!