Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial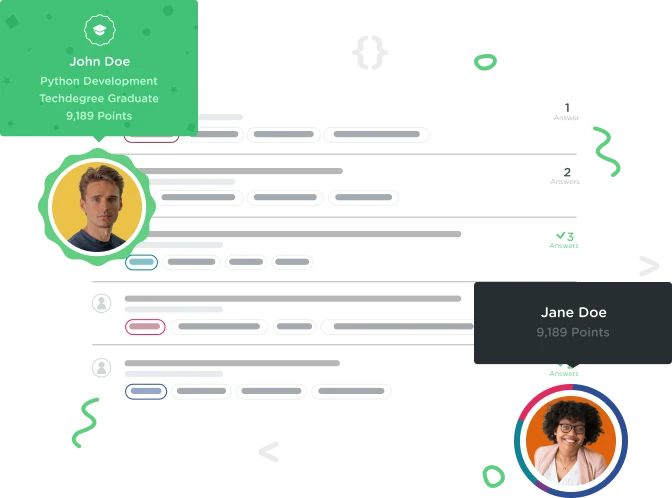
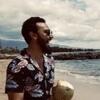
Ali Raza
13,633 PointsHTML & CSS for this Course
Everyone,
I am including the HTML and CSS for this course since Workspace was having issue with the code. I hope it will get fixed but until then use this.
HTML
<!doctype html>
<html lang="en">
<head>
<title></title>
<link href='https://fonts.googleapis.com/css?family=Varela+Round' rel='stylesheet' type='text/css'>
<link href='styles/main.css' rel='stylesheet' type="text/css">
</head>
<body ng-app="todoListApp">
<h1 ng-click="helloWorld()">My TODOs</h1>
<div ng-controller="mainCtrl" class="list">
<div class="item" ng-class="{'editing-item': editing, 'edited': todo.edited}" ng-repeat="todo in todos">
<input ng-model="todo.completed" type="checkbox" />
<label ng-hide="editing" ng-click="helloWorld()">{{todo.name}}</label>
<input ng-change="todo.edited = true" ng-blur="editing = false;" ng-show="editing" ng-model="todo.name" class="editing-label" type="text" />
<div class="actions">
<a href="" ng-click=" editing = !editing">Edit</a>
<a href="" ng-click="helloWorld()">Save</a>
<a href="" class="Delete">delete</a>
</div>
</div>
</div>
<script src="vendor/angular.js" type="text/javascript"></script>
<script src="scripts/app.js" type="text/javascript"></script>
</body>
</html>
CSS
body {
color: #2d3339;
font-family: "Varela Round";
text-align: center;
background: #edeff0;
font-size: 16px;
}
a {
color: #3f8abf;
text-decoration: none;
}
a:hover {
color: #65a1cc;
}
.list {
background: #fff;
width: 80%;
min-width: 500px;
margin: 80px auto 0;
border-top: 40px solid #5a6772;
text-align: left;
}
.list .item {
border-bottom: 2px solid #edeff0;
padding: 17px 0 18px 17px;
}
.list .item label {
padding-left: 5px;
cursor: pointer;
}
.list .item .editing-label {
margin-left: 5px;
font-family: "Varela Round";
border-radius: 2px;
border: 2px solid #a7b9c4;
font-size: 16px;
padding: 15px 0 15px 10px;
width: 60%;
}
.list .item .actions {
float: right;
margin-right: 20px;
}
.list .item .actions .delete {
color: #ed5a5a;
margin-left: 10px;
}
.list .item .actions .delete:hover {
color: #f28888;
}
.list .item.editing .actions {
margin-top: 17px;
}
.list .edited label:after {
content: " edited";
text-transform: uppercase;
color: #a7b9c4;
font-size: 14px;
padding-left: 5px;
}
.list .completed label {
color: #a7b9c4;
text-decoration: line-through;
}
.list .add {
padding: 25px 0 27px 25px;
}
/*
input[type="checkbox"] {
display:none;
}
input[type="checkbox"] + span {
display:inline-block;
width:19px;
height:19px;
margin:-1px 4px 0 0;
vertical-align:middle;
background:url(checkbox-empty.svg) left top no-repeat;
cursor:pointer;
}
input[type="checkbox"]:checked + span {
background:url(checkbox-filled.svg) left top no-repeat;
}*/
Steven Parker
231,275 PointsSteven Parker
231,275 PointsYou did a good job formatting your HTML and CSS code. But you forgot to include the JavaScript component (app.js), or to describe what kind of issue you are having with code.
Also, I noticed you have
DOCTYPE
in lower case.