Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial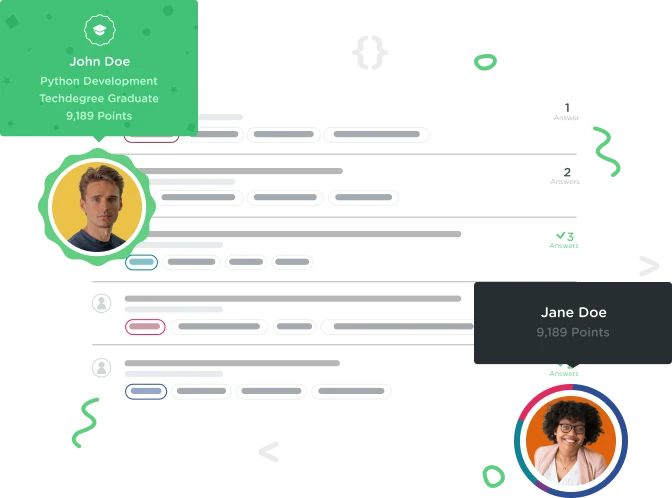

Nicolae Laurentiu
1,538 PointsHy, I don't understand why the code doesn't run
class Fruit:
test = "test"
class Orange(Fruit):
has_pulp = True
def squeeze(self):
return has_pulp
print(Orange().squeeze())
[MOD: added ```python formatting -cf]
2 Answers
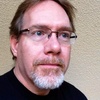
Chris Freeman
Treehouse Moderator 68,423 PointsThe error in running your code is:
Traceback (most recent call last):
File “<string>”, line 12, in <module>
File “<string>”, line 9, in squeeze
NameError: global name ‘has_pulp’ is not defined
To reference the 'has_pulp' attribute of a class instance
, the instance needs to be referenced. The parameter self
is used to point to the current instance. Using self.has_pulp
will fix the error.
Post back if you need more help. Good luck!!!
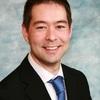
Mark Chesney
11,747 PointsI'm very interested in how this works. I'm normally used to doing OOP like so:
mandarin_orange = Orange()
mandarin_orange.squeeze()
I didn't know it was even possible to do Orange().squeeze()
(of course, if the self.has_pulp
attribute were correctly implemented). Would you call this a method call directly on the class, rather than an instantiated object's method call (as would be mandarin_orange.squeeze()
) ?
feeling kinda confused but kind of learning
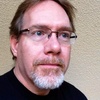
Chris Freeman
Treehouse Moderator 68,423 PointsGood question! The key is in the parens.
The Orange
() in Orange().squeeze()
is creating an instance of the class, then calling the squeeze
method of that instance. The parens call the class to create the instance.
If the parens are removed, then an error is raised because self
doesn’t exist until the class instance wasn’t created. Without parens, it would be calling a classmethod.
Decorating a method with @classmethod
allows access before the instance is created.
class Fruit:
test = "test"
class Orange(Fruit):
has_pulp = True
def squeeze(self):
return self.has_pulp
@classmethod
def squeeze2(cls):
return cls.has_pulp
print(Orange().squeeze())
print(Orange.squeeze2())
# prints
# True
# True