Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial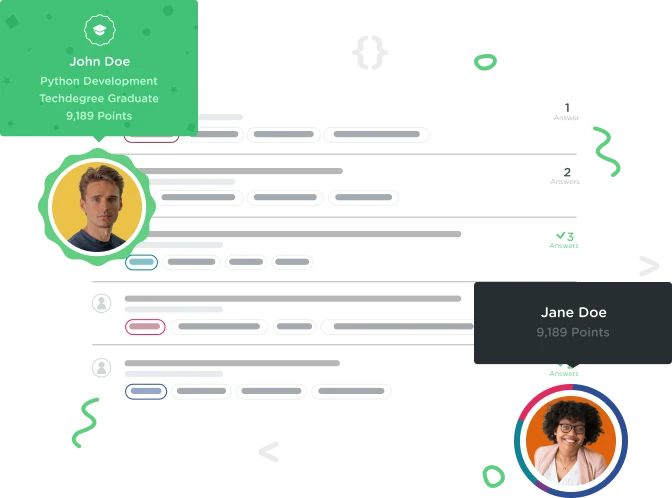

Michael Niers
7,560 PointsI absolutely don't know how to do this: Complete the code by setting the variable listItems to refer to a collection...
Complete the code by setting the variable listItems to refer to a collection. The collection should contain all list items in the <ul> element with the ID of rainbow.
What do I have to do?
var listItems = documentQuerySelectorAll('li:nth-child()');
var colors = ["#C2272D", "#F8931F", "#FFFF01", "#009245", "#0193D9", "#0C04ED", "#612F90"];
for(var i = 0; i < colors.length; i ++) {
listItems[i].style.color = colors[i];
}
<!DOCTYPE html>
<html>
<head>
<title>Rainbow!</title>
</head>
<body>
<ul id="rainbow">
<li>This should be red</li>
<li>This should be orange</li>
<li>This should be yellow</li>
<li>This should be green</li>
<li>This should be blue</li>
<li>This should be indigo</li>
<li>This should be violet</li>
</ul>
<script src="js/app.js"></script>
</body>
</html>
5 Answers
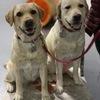
Alex Koumparos
Python Development Techdegree Student 36,887 PointsHi Michael,
We can select an element using code like this (let's imagine that the target element had the ID 'select-me'):
var myElement = document.querySelector('#select-me');
Once we have an element selected, we want to select all its children as a single collection. The challenge provides a link to the MDN documentation, the first line of which provides the following example code:
var children = node.children;
Thus we could use this approach with our code from above as follows:
var myElement = document.querySelector('#select-me');
var children = myElement.children;
Since myElement
is the same object as document.querySelector('#select-me')
, we could replace our reference to the myElement
variable with the selector itself and shrink the two lines of code to just one:
var children = document.querySelector('#select-me').children;
Hope that clears things up.
Cheers
Alex
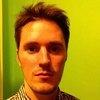
Brendan Whiting
Front End Web Development Techdegree Graduate 84,736 Points1) You need a period between document
and querySelectorAll
2) querySelectorAll
needs to start with a lowercase q. There is an object called document
and querySelectorAll
is a method you call on that object.
3) The argument you need to pass to that method is ul#rainbow li
. I'm not sure how you got to li:nth-child()
. What they want here is "all list items in the <ul> element with the ID of rainbow.".
Let me know if you need more help.

realedwardleung
7,197 Pointsvar listItems = document.querySelectorAll("li");

Lindsey Howard
Courses Plus Student 1,212 Pointslet listItems = document.getElementsByTagName("li");
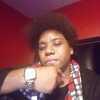
Jamar Sloss
3,463 PointsThanks, your answer is correct.
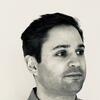
Theo Lufkin
Web Development Techdegree Graduate 15,577 PointsThis is very simple. Took me a few minutes to have the lightbulb above my head turn on... The replies so far on this page do not work. It's easy, you replace querySelector with .getElementsByTag(); like Guil explains in the video before the challenge.