Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial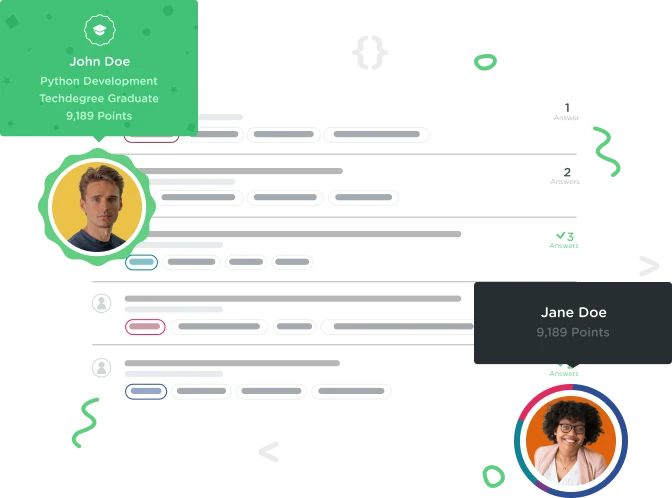
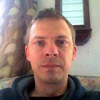
Carl Hart
4,870 PointsI added a little extra code to show number of attempts left....
I added a little extra code to show users the number of attempts they have left, this was the best I could do with my limited knowledge (only just started learning Python), would this be viewed as an efficient/valid/clean method, or is there a better way? (see code below)
import sys
MASTER_PASSWORD = 'letmein'
password = input("Please enter your password: ")
attempt_count = 1
tries_left = 3 # Defined this variable to store number of password attempts left
while password != MASTER_PASSWORD:
if attempt_count > 3:
sys.exit("Too many attempts")
password = input("Invalid password, you have {} attempts remaining, try again: ".format(tries_left))
attempt_count += 1
tries_left = 4 - attempt_count # An operation on the 'tries_left' variable to derive attempts countdown value from 'attempt_count'
print("you are now logged in!")
2 Answers
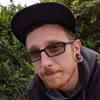
Travis Alstrand
Treehouse Project ReviewerHey Carl Hart ! 👋
Nice addition!! 👏
I haven't tested it or anything, but it looks like a nice function you've created here! How is it working for you?
I'm no pro either, but it looks great to me! I'm sure, as always, you'll keep learning more new things / approaches and come up with ways to refactor things in the future. Great work! 😃
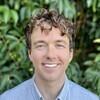
Asher Orr
Python Development Techdegree Graduate 9,410 PointsNice work, Carl Hart!
I posted my solution below. It has an additional line, but I think it's a little easier to read.
You can be the judge of that, though 😃
import sys
password_list = []
while True:
MASTER_PASSWORD = 'letmein'
password = input("Please enter your password: ")
password_list.append(password)
if len(password_list) == 3:
sys.exit("No valid passwords entered. Maximum number of attempts reached. The program is closing now.")
elif password != MASTER_PASSWORD:
re_try = input(f'Invalid password, you have {3 - len(password_list)} attempts left. Press enter. ')
else:
break
print("You are now logged in.")
Let me know if you have any questions!
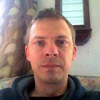
Carl Hart
4,870 PointsHi Asher, thank's for your feedback, like I said I am very much a noob, so I could only go on what I know. While I think I partly understand what you did in your version of the code (yes it looks cleaner, thanks!) can you confirm what ' password_list.append(password)' is doing? I could be wrong but it looks like by appending the input variable to password_list, the 'len(password_list)' returns the number of entries which can then be compared to the max count of 3, hence 'if len(password_list) == 3:' the program exits? Hope that makes sense!
This also means it would be very easy to set the maximum number of tries in a constant before the while loop, so:
import sys
MAX_ATTEMPTS = 3
password_list = []
while True:
MASTER_PASSWORD = 'letmein'
password = input("Please enter your password: ")
password_list.append(password)
if len(password_list) == MAX_ATTEMPTS:
sys.exit("No valid passwords entered. Maximum number of attempts reached. The program is closing now.")
elif password != MASTER_PASSWORD:
re_try = input(f'Invalid password, you have {MAX_ATTEMPTS - len(password_list)} attempts left. Press enter. ')
else:
break
print("You are now logged in.")
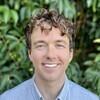
Asher Orr
Python Development Techdegree Graduate 9,410 PointsYou're welcome Carl Hart! No worries on being a beginner. Asking for help and seeing a new way of solving a problem is one of the best learning methods, IMO. I'm glad you're doing it! 😊
Q: Can you confirm what ' password_list.append(password)' is doing? I could be wrong, but it looks like by appending the input variable to password_list, the 'len(password_list)' returns the number of entries which can then be compared to the max count of 3, hence 'if len(password_list) == 3:' the program exits? Hope that makes sense!
You are correct. The user's input (stored in the "password" variable) is added to password_list. Calling the len() function on the list returns the number of elements within the list.
The program will exit when the number of items in password_list reaches 3, as you mentioned. You can definitely add the MAX_ATTEMPTS variable!