Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial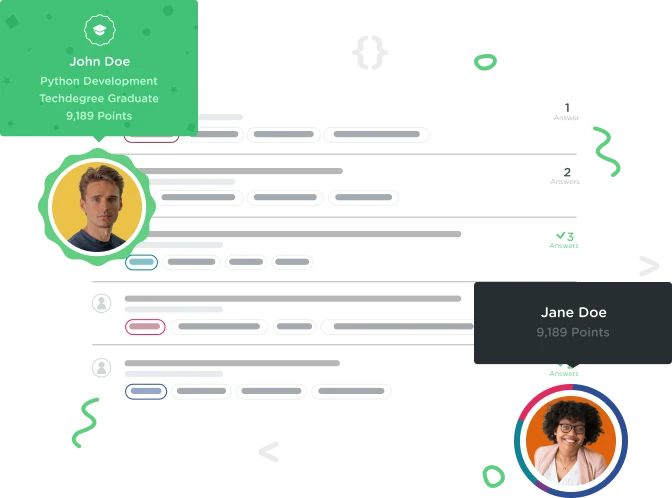
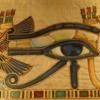
Gregory James
6,537 PointsI already pass this challenge but I am trying to gain a better understanding
How can I unpack this when calling the function? Do I need to twik the function itself? I keep getting a name not defined when i used this format.
def favorite_food(dict):
return "Hi, I'm {name} and I love to eat {food}!".format()
favorite_food(**{name:"Greg",food:"vegan"})
1 Answer

kyle kitlinski
5,619 PointsHello Greg, You are close but there are a few issues:
1) Unlike javascript, in python the dictionary keys must be strings favorite_food(**{"name": "Greg", "food": "vegan"})
2) the function takes in a dict, not name and food. So there's 2 ways you can fix this.
a) unpack the dict outside of the function and take in name and food as arguments
def favorite_food(name, food):
return f"Hi, I'm {name} and I love to eat {food}!"
print(favorite_food(**{"name":"Greg","food":"vegan"}))
b) take in the dict as you are, but unpack it inside the function:
def favorite_food(dict):
return "Hi, I'm {name} and I love to eat {food}!".format(**dict)
print(favorite_food({"name":"Greg","food":"vegan"}))
I think option B is more of what you're looking for since you're practicing dictionaries though
edit for clarification: In your sample you are spreading the dictionary {"name":"Greg","food":"vegan"} and calling the function. This is effectively the same as
name = "Greg"
food = "vegan"
favorite_food(name, food)
# this is 2 arguments but you only want 1 dict as an arg
# then with "Hi, I'm {name} and I love to eat {food}!".format()
# you aren't passing anything to the format function, so the name and food
# aren't defined, so if you unpack the dictionary there, you are passing name and food to the f' string
# "Hi, I'm {name} and I love to eat {food}!".format(**dict)
Hope this helps!