Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial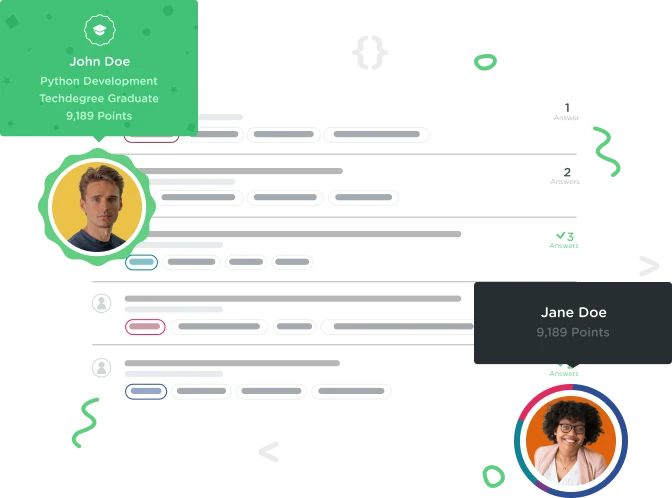
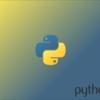
Kevin Faust
15,353 Pointsi always am never able to do these challenges....here's my fail code. any help would be really appreciated
var questions = [
['How many states are in the United States?', '50'],
['How many continents are there?', '7'],
['How many legs does an insect have?', '6']
];
var correctAnswers = 0;
var question;
var answer;
var response;
var html;
var html1;
var html2;
var questionsCorrect=[];
var questionsWrong=[];
var listedCorrect="";
var listedWrong="";
function print(message) {
var outputDiv = document.getElementById('output');
outputDiv.innerHTML = message;
}
for (var i = 0; i < questions.length; i += 1) {
question=questions[i][0];
response=prompt(question);
answer=questions[i][1];
if (response===answer) {
correctAnswers +=1;
questionsCorrect.push(question);
}
else {
questionsWrong.push(question);
}
}
//display each array in ordered list form
listedCorrect += "<ol>";
for (var i=0; i<questionsCorrect.length; i++) {
listedCorrect += "<li>" + questionsCorrect[i] + "</li>";
}
listedCorrect += "</ol>";
listedWrong += "<ol>";
for (var i=0; i<questionsWrong.length; i++) {
listedWrong += "<li>" + questionsWrong[i] + "</li>";
}
listedWrong += "</ol>";
html = "You got " + correctAnswers + " question(s) right."
print(html);
html1 = "You got the following questions correct: " + listedCorrect;
print(html1);
html2 = "You got the following questions incorrect: " + listedWrong;
print(html2);
so when this code runs, it only shows the the questions incorrect and the amount of questions that i answered correctly. for some reason the questions that are answered correct dont show up
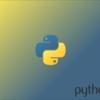
Kevin Faust
15,353 Pointsok i put the 3 ticks
1 Answer
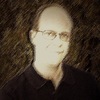
Jason Anders
Treehouse Moderator 145,858 PointsHey Kevin,
The reason it is only printing which questions you got wrong is because that is the last call of the print function
you do. Every time the function is called, it writes to the #output div. The problem is when you call it again, it just overwrites what was there before, and then a third time for the incorrect answers.
Here is one (of many ways) you can correct this:
html = "You got " + correctAnswers + " question(s) right. </br></br>";
html += "You got the following questions correct: " + listedCorrect + "</br>";
html += "You got the following questions incorrect: " + listedWrong;
print(html);
Hope this helps.
Also, a suggestion... try to find ways to not use that many variables. Remember, DRY coding is the best. This is something that comes with practice, so don't worry too much about it now, I just wanted to give you an example, which I hope will help you with your JS journey.
Example: You could use
for (var i = 0; i < questions.length; i += 1) {
response=prompt(questions[i][0]);
if (response===questions[i][1]) {
correctAnswers +=1;
questionsCorrect.push(questions[i][0]);
} else {
questionsWrong.push(questions[i][0]);
}
}
instead of
for (var i = 0; i < questions.length; i += 1) {
question=questions[i][0];
response=prompt(question);
answer=questions[i][1];
if (response===answer) {
correctAnswers +=1;
questionsCorrect.push(question);
}
else {
questionsWrong.push(question);
}
}
Hope this all makes sense and Keep Coding! :)
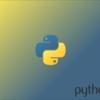
Kevin Faust
15,353 PointsHey Jason,
Thanks for your answer. Everything is good now :) Cheers!
Chad Donohue
5,657 PointsChad Donohue
5,657 PointsPlace three back ticks (`) before and after your code. This formats it so that others can view your code easier.