Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial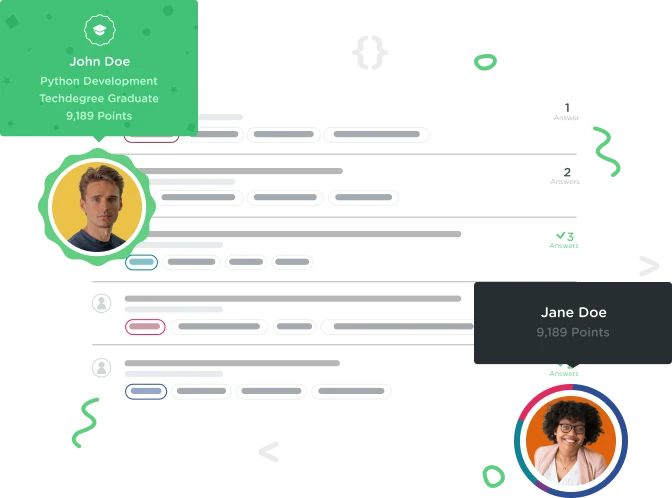

Bradley Isenbek
2,629 PointsI am at a loss with this quiz. I create typealias BlogPostCompletion = (NSData!, NSURLResponse!, NSError!) -> Void
What am I doing wrong?
import Foundation
typealias BlogPostCompletion = (NSData!, NSURLResponse!, NSError!) -> Void
// Add your code below
func fetchTreehouseBlogPosts (completion: BlogPostCompletion) {
}
import Foundation
typealias BlogPostCompletion = (NSData!, NSURLResponse!, NSError!) -> Void
// Add your code below
func fetchTreehouseBlogPosts (completion: BlogPostCompletion) {
}
3 Answers
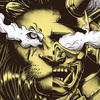
Joshua C
51,696 PointsYou're really close!
You forgot to enclose the typealias result in parentheses (including the "Void" at the end):
typealias BlogPostCompletion = ((NSData!, NSURLResponse!, NSError!) -> Void)
// Add your code below
func fetchTreehouseBlogPosts (completion: BlogPostCompletion) {
}
Alp Eren Can
25,230 PointsHi Brian,
In the video, Pasan didn't enclose the typealias result in parentheses but it seems to be working just fine. Can you think of a reason for this?
Thanks.

Michael Zaro
13,196 PointsI'd like to know the same thing as Alp Eren Can... or is this just an instance of another Treehouse objective that is being more strict that it should be? Because I run into those a lot.

Bradley Isenbek
2,629 PointsBrian,
Thank you my friend! You are a martial artist and an iOS Ninja ...
-Brad

james white
78,399 PointsYes, thanks Brian. I was over thinking the code.
For those who find Brian's code helpful towards getting past task 1 of 2 for the objective, I would recommend this helpful thread if you get stuck on task 2 of 2 for the same objective:
https://teamtreehouse.com/forum/cant-return-results-to-method-via-completion-handler
james white
78,399 Pointsjames white
78,399 PointsJust want to provide a link to the objective:
http://teamtreehouse.com/library/build-a-weather-app-with-swift-2/managing-complexity/callback-methods-with-closures
Here's the objective:
There is another forum post about this (which doesn't have many responses): https://teamtreehouse.com/forum/whats-wrong-on-this-syntax-in-swift
Notice though it says:
I don't think this code has anything asynchronous about it.
I found this swift code:
..on this StackOverFlow page:
http://stackoverflow.com/questions/27720021/swift-cant-send-urlrequest-at-all
maybe there should be some code like that?
It's similar to code on this page:
https://gist.github.com/cmoulton/01fdd4fe2c2e9c8195e1
However, this code would have to be adapted to use the fetchTreehouseBlogPosts method somehow..
I would also note there is a TeamTreehouse blog post about making asynchronous network requests in Swift:
http://blog.teamtreehouse.com/making-network-request-swift
However, none of the code in that blog post seems to pass.
Looking at the NetworkOperations code in the course Downloads "Stormy3V2" zip file for the course,
I found a typealias line so I modified that code to conform with the questions and got:
However it didn't like that code either (although the output.html showed no errors), it still gave: