Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial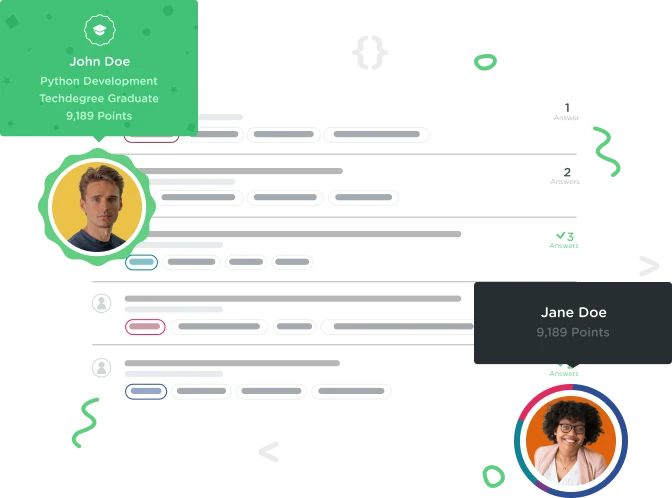

Mike Sagafi
2,128 PointsI am at loss. The defined variables are constants. If I create a custom method then I can't update them
isn't this the way todo it?
struct RGBColor { let red: Double let green: Double let blue: Double let alpha: Double
let description: String
// Add your code below
init (red: Double, green: Double, blue: Double, alpha: Double, description: String) {
self.red = red
self.green = green
self.blue = blue
self.alpha = alpha
self.description = "red: \(red), green: \(green), blue: \(blue), alpha: \(alpha)"
}
}
struct RGBColor {
let red: Double
let green: Double
let blue: Double
let alpha: Double
let description: String
// Add your code below
init (red: Double, green: Double, blue: Double, alpha: Double, description: String) {
self.red = red
self.green = green
self.blue = blue
self.alpha = alpha
self.description = "red: \(red), green: \(green), blue: \(blue), alpha: \(alpha)"
}
}
2 Answers

Joseph Kato
35,340 PointsHi Mike,
It looks like the issue is that your initializer method is expecting to be passed a value (that isn't actually used) for description
:
init (red: Double, green: Double, blue: Double, alpha: Double, description: String) {
Consider the following example:
struct Point {
let x: Int
let y: Int
let description: String
init (x: Int, y: Int, description: String) {
// Note: we don't use the `description` argument here...
self.x = x
self.y = y
self.description = "x: \(x), y: \(y)"
}
}
Given this definition of a Point
, we can't create our own by simply passing values for x
and y
:
var myPoint = Point(x: 1, y: 2) // "ERROR: missing argument for parameter 'description' in call"
So, we'd have to do something like this:
var myPoint = Point(x: 1, y: 2, description: "this isn't used!")
But we don't want to have to do that every time, so we re-write our initializer method as:
init (x: Int, y: Int) {
self.x = x
self.y = y
self.description = "x: \(x), y: \(y)"
}
// We can now say `var myPoint = Point(x: 1, y: 2)`!

Florian Thompson
Courses Plus Student 18,609 PointsHi there,
your properties need to be declared as var
in order for you to override them.
You would go with let
if you would give them an initial value.
if you want to avoid them to be modified you might want to change the access level. For example:
struct RGBColor {
private var description: String
}
That way you can't change the value from outside the struct. If you would want to, you can write a helper method which needs to be marked as @mutating func
I hope this helped.