Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial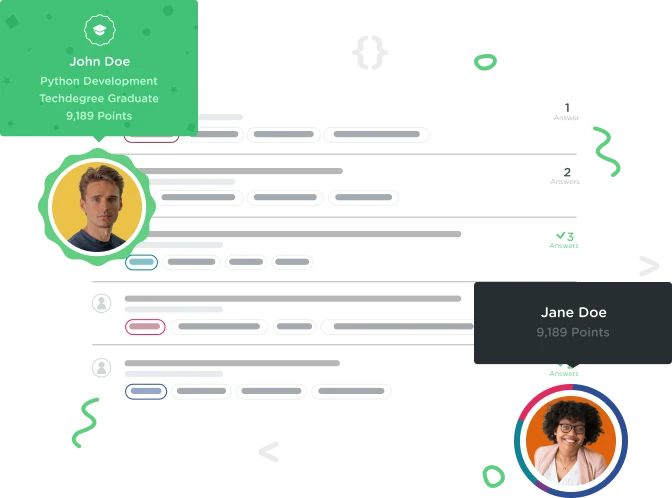

Ragesh Kanagaraj
3,637 PointsI am completely confused with this customer constructor concepts in this current tutorial
Can anyone explain me this concept of custom constructor and why are we using it ? I have decent knowledge on java. But this tutorial seems to be very confusing for me and I am not able to understand why we are creating classes for story page choice and calling the constructors.
1 Answer
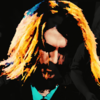
Justin Horner
Treehouse Guest TeacherHello Ragesh,
There are several reasons why one might add custom constructors to a class. In this specific example, custom constructors are used to make the model simple and easy to construct.
So think of a class as a blueprint for a house. The class has the design layout for what an object instance will look like when constructed. Every class has a default constructor that has no parameters. This default constructor is included by Java on your behalf so you don't have to create a parameterless constructor for every class you create.
Using a custom constructor allows you to construct the class with the default values you want to supply. So now imagine what it would be like to create and setup the objects without the convenience of the custom constructors that were created in the video. You would have to instantiate the object with the default constructor and then call several methods immediately following the creation of the object like this.
private void MethodA() {
ClassA classA = new ClassA();
classA.setProperty1("value 1");
classA.setProperty2(true);
classA.setProperty3(6);
classA.setProperty4("value 4");
...
ClassB classB = new ClassB();
classB.setProperty1("value 1");
classB.setProperty2("value 2");
classB.setProperty3(true);
classB.setProperty4(23);
...
}
Not very elegant, in my opinion. If we add custom constructors to ClassA and ClassB we'll be able to accept values to be set on member variables during the construction of the object. It would look like this.
private void MethodA() {
ClassA classA = new ClassA("value 1", true, 6, "value 4");
ClassB classB = new ClassB("value 1", "value 2", true, 23);
}
A lot nicer to work with, right? Our API is now simpler and easier to use. The custom constructor simply allows you to define what the defaults are instead of the class making assumptions and depending on the user of the class to call all the right set methods to properly configure the class as needed.
I hope this helps.