Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial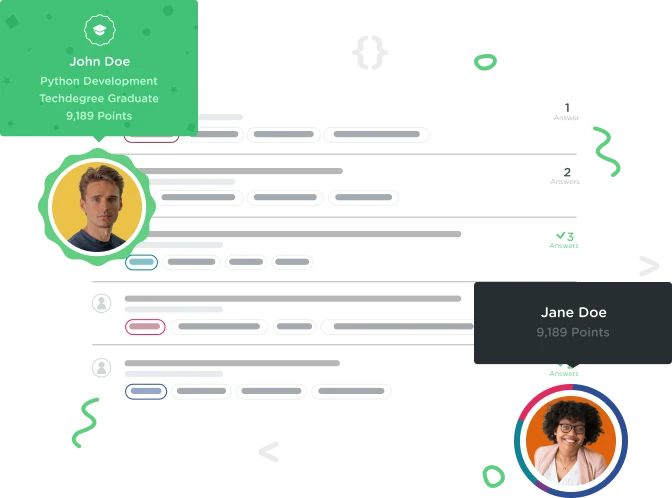

matthew comeaux
1,449 PointsI am completely lost! I don't understand the program.
How does the 'for loop' in the 'while loop' work. I am struggling to understand how the 'if statement' works in this. It seems like if the 'search variable' was different from the 'i variable' in the 'for loop', then the program wouldn't run. For instance if the loop ran the first time 'i' would equal '0' and lets say the search was for the third student ( i = 2) then nothing would happen because 0 /= 2. Why isn't this so? Also, how does the 'if statement' in the 'for loop' know what 'student.name' even is? If the questions are unclear please feel free to ask for clarification. Any help would be greatly appreciated.
var message = ''; var student; var search;
function print(message) { var outputDiv = document.getElementById('output'); outputDiv.innerHTML = message; }
function getStudentReport( student ) { var report= '<h2>Student: ' + student.name + '</h2>'; report += '<p>Track: ' + student.track + '</p>'; report += '<p>Points: ' + student.points + '</p>'; report += '<p>Achievements: ' + student.achievements + '</p>'; return report; }
while (true) { search = prompt('To search student records: type a name [Jody] (or type "quit" to exit program)'); if (search === null || search.toLowerCase() === 'quit'){ break; } for (var i = 0; i < students.length; i += 1) { student = students[i]; if (student.name.toLowerCase() === search.toLowerCase()) { message = getStudentReport(student) print(message) } };
1 Answer

andren
28,558 PointsIt's true that if you search for a student with an index of 2 for example then the for
loop would not find it the first time it ran, but that's not an issue. The point of a loop is that it runs multiple times. The second time the loop runs i
would equal 1, and then the third time it would equal 2. At that point it would pull out the right student and the code would run as intended.
The line:
student = students[i];
Assigns one of the students to the student
variable, that's why student.name
can be used to pull out the name of a student. It will pull out the first student the first loop, the second student the second loop and so on. If the name you searched for match the name of the student pulled out then the code within the if
statement runs, if not then the loop just starts its next iteration as normal.
Edit:
Here is a version of the for
loop with some comments added:
// Loop once for every student in the students array
for (var i = 0; i < students.length; i += 1) {
// Assign a student to the student variable
student = students[i];
// See if the current student's name matches the one that is searched for.
// If that is the case run the code within
if (student.name.toLowerCase() === search.toLowerCase()) {
message = getStudentReport(student)
print(message)
}
// If that is not the case then the loop will just move on to its next iteration
};
matthew comeaux
1,449 Pointsmatthew comeaux
1,449 PointsThank you for your help!