Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial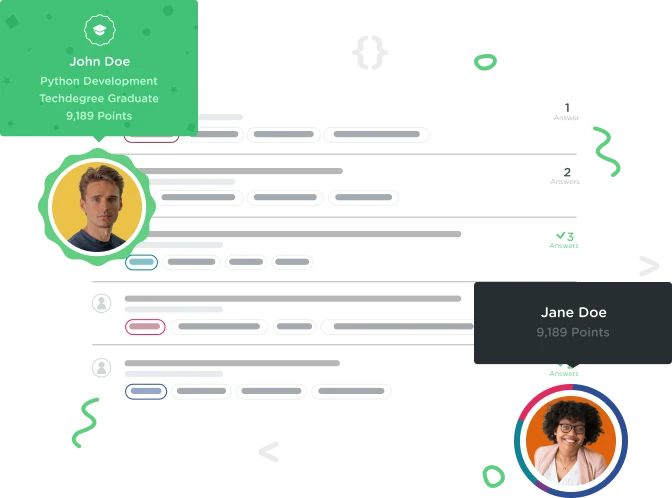
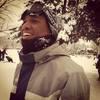
King Penson II
16,413 PointsI am completely stumped at this Contains? method
I've tried everything to if/else statements to the current code I have now. I fooled around with the include? method and got it to return a boolean in irb, I just can't figure out this challenge.
class TodoList
attr_reader :name, :todo_items
def initialize(name)
@name = name
@todo_items = []
end
def add_item(name)
todo_items.push(TodoItem.new(name))
end
def contains?(name)
todo_items.include?()
end
def find_index(name)
index = 0
found = false
todo_items.each do |item|
found = true if item.name == name
break if found
index += 1
end
if found
return index
else
return nil
end
end
end
3 Answers
William Li
Courses Plus Student 26,868 PointsOr, you can approach it this way, make use of the find_index method
def contains?(name)
!!find_index(name) # convert find_index(name) to boolean value
end
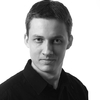
Maciej Czuchnowski
36,441 PointsYou could use the find_index method and do something like this:
def contains?(name)
if find_index(name)
return true
else
return false
end
end
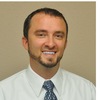
Paul Stettnisch
10,712 PointsThank you Maciej. While the other two responses seem like they would work, they are not what was taught in the previous video. I believe this solution is what students can be expected to be able to use this point in their learning.
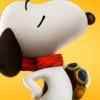
Salman Akram
Courses Plus Student 40,065 PointsHi King,
Other way you can try is .any? method to see if the block returns a value of true with the name arguments.
def contains?(name)
todo_items.any? { |item_array| item_array.name==name }
end
More information on Ruby Doc & Block examples
Hope that helps.
King Penson II
16,413 PointsKing Penson II
16,413 PointsSorry for the late response, this solution worked. I honestly feel like I was trying too hard.