Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial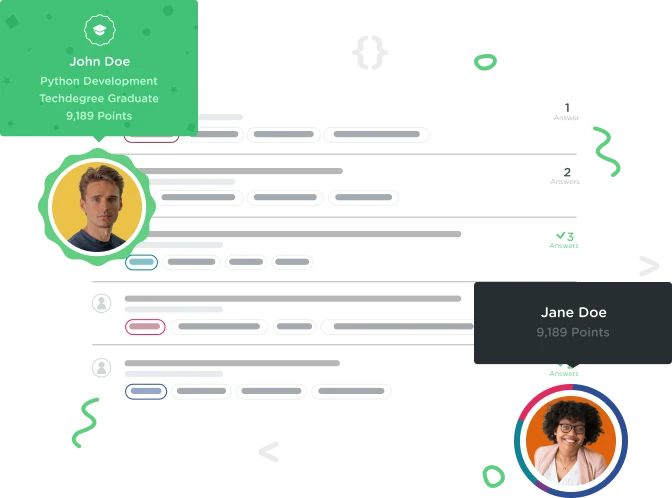

Sabine Lazuhina
2,334 PointsI am confused about "self" and use of other new variables in methods.
I am confused about "self" and use of other new variables in methods.
class Student:
name = "Sabine"
def praise(self):
return "You inspire me, {}".format(self.name)
def reassurance(self):
return "Chin up, {}. You'll get it next time!".format(self.name)
def feedback(self,grade):
grade = int(input("Your grade is: "))
if grade > 50:
return praise
else:
return reasurance
1 Answer

lemelleio
10,874 PointsHey Sabine,
I struggled with this for a while because it was such an abstract way of thinking at first but I eventually let it simmer in my mind and after working with classes and objects enough I began to understand it.
You can think of it like this:
a class is like writing a list of things that something can do. Methods are the things in that list. Methods are just functions.
When you write a function that takes an argument you are saying that you want to pass some variable into the function, perform some type of operation, and then return the output:
def addingfunc(thingiwanttodostuffto):
thingiwanttodostuffto += 1
return thingiwanttodostuffto
in order to understand self and class variables we have to think about how objects work. An object is basically a collection of data that sits in a computers memory and it's named with a reference number(called a memory address) in order to be retrieved later by the cpu.
so in best practice of programming we want to minimize typing repetitive things so for example say I was working on a game and I wanted it to have multiple characters. I wouldn't want to write a separate class for each of those objects because I would be writing much of the same methods on each class, so why not use one class and make each character an instance of that class.
So what if each of the characters had different colored hats? How would the computer know which object(instance of my character class) had which colored hat? Essentially I have three copies of the same class sitting in their respective spots in memory right? That is the purpose of self. It provides a self reference of each instance of the class. it provides identification(memory reference) of which class instance(object) you are working with.
class variables are how you can provide each instance(object) of your base class with the same initial value.
Say I wanted each of my characters to start with 100 health points, but also allow each instance to change that value based on if they get it in the game or not.
Hopefully that helps, if anyone more experienced sees any inaccuracy here, please correct me.