Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial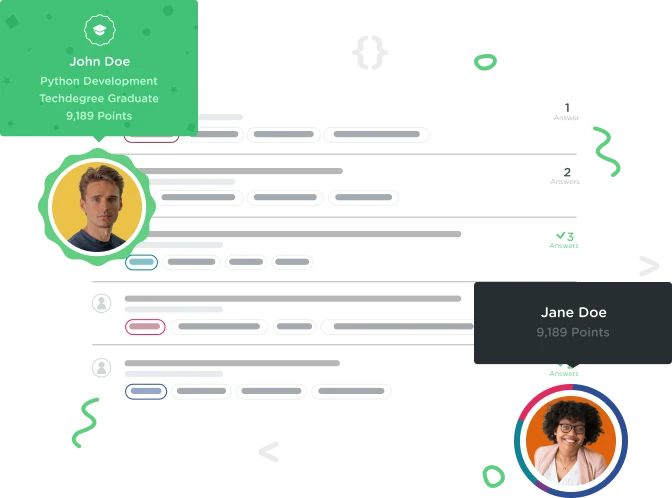
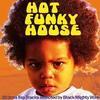
Jeff Burk
5,215 PointsI am confused on the "out" keyword in the TryParse method.
TryParse(this, out that) always needs an out keyword? Is "out" used elsewhere in other methods? Please enlighten me.
2 Answers

andren
28,558 Pointsout
is a keyword that modifies how the argument is passed into the method, and it is considered part of the method's signature. Meaning that the keyword has to be included both when the method is defined and when it is called.
It causes the argument to be passed by reference, and not by value as is usually the case.
Take this code for example:
using System;
class MainClass {
public static void Main()
{
int var1 = 0;
SetVariableTo5(var1);
Console.WriteLine(var1);
}
public static void SetVariableTo5(int a)
{
a = 5;
}
}
What would you expect the above code to print? Well the answer is 0, because while you pass var1
into SetVariableTo5
that only passed the value of var1
, not the actual variable so changing the value of a
won't do anything to var1
.
With this code however:
using System;
class MainClass {
public static void Main()
{
int var1 = 0;
SetVariableTo5(out var1);
Console.WriteLine(var1);
}
public static void SetVariableTo5(out int a)
{
a = 5;
}
}
Things are different. With this code 5 will in fact be printed. And this is due to the fact that when out
is used you pass in the actual variable to the function, not just its value. Which means any changes done to it within the method will affect the variable that was passed in as an argument.
It's worth mentioning that there is also a keyword called ref
that works pretty much identically to out
, with the main difference being that ref
requires that the variable has to have a value assigned to it. out
accepts empty variables.
So code like this:
using System;
class MainClass {
public static void Main()
{
int var1;
SetVariableTo5(out var1);
Console.WriteLine(var1);
}
public static void SetVariableTo5(out int a)
{
a = 5;
}
}
Is valid with the out
keyword but not with the ref
keyword. While the code shown in the other example I provided would work with either out
or ref
.

benovarghese
2,059 PointsWhat is the benefit of using the keyword out (in the example you provided) instead of the following? Does it affect performance or memory in any way? for larger applications.
using System;
class MainClass { public static void Main() { int var1 = 0; int var2 = SetVariableTo5(var1); Console.WriteLine(var2); }
public static int SetVariableTo5(int a) { return a = 5; } }
Jeff Burk
5,215 PointsJeff Burk
5,215 PointsAhh. Cool. That makes sense. I'll have to try that out. Thanks!!
chazber
4,873 Pointschazber
4,873 PointsPerfect explanation.