Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial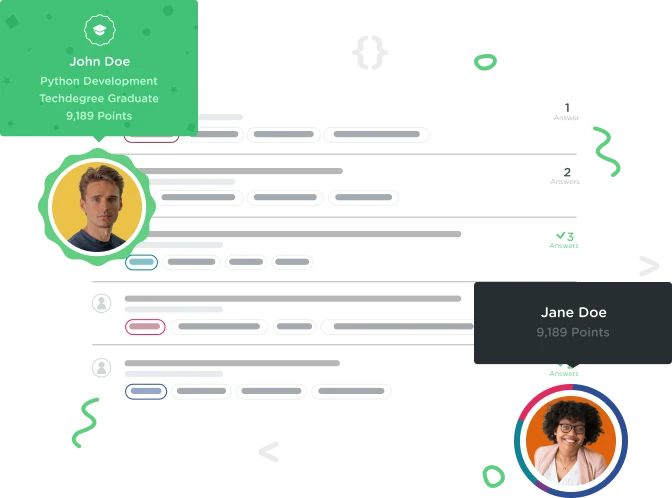

Cody Adkins
7,260 PointsI am curious to find out how you solved your assignment at the end of this video?
Looking forward to hearing back.
5 Answers

Greg Kaleka
39,021 PointsHey Cody,
Here's my back-of-the-napkin-not-even-typed-into-xcode-so-very-possibly-buggy solution. I modified the method to return a named tuple.
func getLarger <T: Comparable> (valueA: T, valueB: T) -> (largestValue: T, valuesAreEqual: Bool) {
if valueA > valueB {
return (largestValue: valueA, valuesAreEqual: false)
} else if valueA == valueB {
return (largestValue: valueA, valuesAreEqual: true)
} else {
return (largestValue: valueB, valuesAreEqual: false)
}
}
Cheers!
-Greg
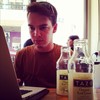
Alexander Batalov
21,887 PointsHow about this
func getLarger<T: Comparable>(a: T, b: T) -> (value: T, isEqual: Bool) {
return (a > b ? a : b, a == b)
}

Greg Kaleka
39,021 PointsNice. I'd add an extra set of parens for clarity:
func getLarger<T: Comparable>(a: T, b: T) -> (value: T, isEqual: Bool) {
return ( (a > b ? a : b) , (a == b) )
}
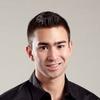
Clayton Cohn
15,715 PointsThat's the way I did it too...nice and succinct.
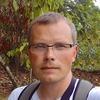
Ivan Kazakov
Courses Plus Student 43,317 PointsChange the return type to Optional and return nil in case of equality. Which syntactically means that none of them is larger than the other.

Kevin Murphy
24,380 PointsMy thoughts as well Ivan. I had made the return value Optional but had a long form if-else. Taking inspiration from the ternary version, refactored into:
func getLargerValue<T: Comparable>(val1: T, val2: T) -> (largerVal: T?, areEqual: Bool) {
return ( (val1 != val2 ? val1 > val2 ? val1 : val2 : nil), (val1 == val2) )
}
"First check if val1 is not equal to val2; If it's not, then evaluate if val1 is greater than val2. If val1 is greater than val2 return val1 otherwise return val2; lastly, the "else" of the outer condition - If val1 is equal to val2, then return nil"

Greg Kaleka
39,021 PointsMaybe... it depends what you want to do with the value. You may be using it for something. If the return value is nil, you would just have to pick one anyway. Again, it depends on the purpose.

Alistair Cooper
Courses Plus Student 7,020 PointsCool.
My version with some string action:
func getLarger <T: Comparable> (valueA: T, valueB: T) -> (largerValue: T, result: String){
if valueA == valueB {
return (valueA, "The two values are equal")
} else if valueA > valueB {
return (valueA, "Value A is larger")
} else {
return (valueB, "Value B is larger")
}
}
var result = getLarger(42, valueB: 42)
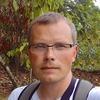
Ivan Kazakov
Courses Plus Student 43,317 PointsHello Kevin, looks nice. Though my 'ternary' version would look like this:
func getLarger <T: Comparable> (valueA: T, valueB: T) -> T? {
return valueA > valueB ? valueA : (valueB > valueA ? valueB : nil)
}
But i'd say using ternary operation within another ternary operation makes code hardly readable and I'd prefer if-else

Kevin Murphy
24,380 PointsHi Ivan, thanks for sharing, great way to tackle. I agree though, readability definitely becomes a factor, and prefer the if-else as well.