Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial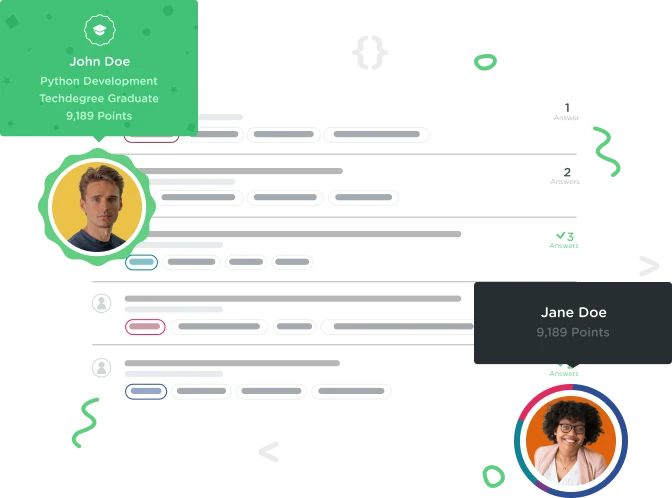
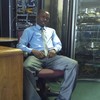
MUZ140698 Kudakwashe Gwaindepi
4,150 Pointsi am failing to run app on phone
im getting the following error message
gradle sync failed, please fix your project and try again
and also this
Waiting for device. Target device: sony-d2403-ZH8004DCGH Uploading file local path: C:\Users\kgwaindepi\AndroidStudioProjects\Funfacts\app\build\outputs\apk\app-debug.apk remote path: /data/local/tmp/kayg.funfacts Local path doesn't exist.
8 Answers

jason phillips
18,131 Pointsahh ok I believe this line is causing you issue:
txtfact.setText("");
try this instead
txtfact.setText(mFactBook.getFact());

jason phillips
18,131 PointsCan you post the build.gradle file?
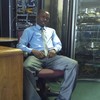
MUZ140698 Kudakwashe Gwaindepi
4,150 Pointswhere do i get that file jason?

jason phillips
18,131 Pointsit should be /app/build.gradle
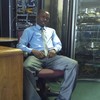
MUZ140698 Kudakwashe Gwaindepi
4,150 Pointsok i got it
here it is:
apply plugin: 'com.android.application'
android { compileSdkVersion 21 buildToolsVersion "21.1.2"
defaultConfig {
applicationId "kayg.funfacts"
minSdkVersion 14
targetSdkVersion 21
versionCode 1
versionName "1.0"
}
buildTypes {
release {
minifyEnabled false
proguardFiles getDefaultProguardFile('proguard-android.txt'), 'proguard-rules.pro'
}
}
}
dependencies { compile fileTree(dir: 'libs', include: ['*.jar']) compile 'com.android.support:appcompat-v7:22.0.0' }

jason phillips
18,131 Pointsthat file looks fine to me. Do you have a github account? My guess is that because gradle can't sync it won't copy the app to the emulator. Sometimes its easier to run down the issue when you have a full project to look at. If you put it up on github or bitbucket I can grab the whole thing and take a look.
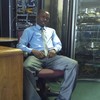
MUZ140698 Kudakwashe Gwaindepi
4,150 Pointshow do i do that, i have just created a github account

jason phillips
18,131 Pointsyou need to install git on your computer. you can find instructions here: http://msysgit.github.io/
Git is unobtrusive and a good thing to have installed, even on windows, for collaboration.
next open up a command prompt and change the directory to your project directory
git init
Next on the github site there is a button on the right side of the page that says create repository, click that. After you name your new repository it will take you to a page basic how to start kind of a page
look for this section "…or push an existing repository from the command line"
these are the commands you will need to run in the command prompt so it will be something like this:
git remote add origin https://github.com/{username}/{repository_title}.git
git push -u origin master
after it has been pushed to github, I will be able to grab it and take a look. I know this seems like a lot, but git seems to install fairly easy. If you run into issue please contact me at jphillips05@gmail.com
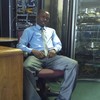
MUZ140698 Kudakwashe Gwaindepi
4,150 Pointshello jason, this morning i tried cleaning my project and i succeeded, and i managed to run it on my phone, thanks to you but now i am facing a minor problem, its displaying only one fun fact, when i click next fact, its displaying nothing, only the layout colors are changing, probably meaning that my colorwheel is working fine, but my factbook is not
here is my layout code
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:paddingLeft="@dimen/activity_horizontal_margin" android:paddingRight="@dimen/activity_horizontal_margin" android:paddingTop="@dimen/activity_vertical_margin" android:paddingBottom="@dimen/activity_vertical_margin" tools:context=".FunfactsActivity" android:background="#ff51fffa" android:id="@+id/relativeLayout">
<TextView android:text="DID YOU KNOW?" android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignParentTop="true"
android:layout_centerHorizontal="true"
android:textColor="#801220ff"
android:textColorHint="#ff70f0ff"
android:textSize="30sp"
android:id="@+id/txtqsn" />
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="ants strecth when they wake up in the morning"
android:id="@+id/txtfact"
android:layout_centerVertical="true"
android:layout_alignParentLeft="true"
android:layout_alignParentStart="true"
android:textColor="#ff1117ff"
android:textSize="25sp" />
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Next fun fact"
android:id="@+id/btnnext"
android:layout_alignParentBottom="true"
android:layout_centerHorizontal="true"
android:textSize="24sp"
android:textColor="#ff1826ff" />
</RelativeLayout>
and here is my factbook code
package kayg.funfacts;
import java.util.Random;
/**
-
Created by kgwaindepi on 4/11/2015. */ public class FactBook {
//member variables public String[] mFacts = { "Ants stretch when they wake up in the morning.", "Ostriches can run faster than horses.", "Olympic gold medals are actually made mostly of silver.", "You are born with 300 bones; by the time you are an adult you will have 206.", "It takes about 8 minutes for light from the Sun to reach Earth.", "Some bamboo plants can grow almost a meter in just one day.", "The state of Florida is bigger than England.", "Some penguins can leap 2-3 meters out of the water.", "On average, it takes 66 days to form a new habit.", "Mammoths still walked the earth when the Great Pyramid was being built." };
public String getFact () {
String fact = ""; //randomly select a fact Random randomGenerator = new Random();// construct a new random number generator int randomnumber = randomGenerator.nextInt(mFacts.length); fact = mFacts[randomnumber]; return fact;
} //abilities or method
}

jason phillips
18,131 Pointscan you post your MainActivity.java or it might be named FunFactsActivity.java FIle
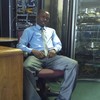
MUZ140698 Kudakwashe Gwaindepi
4,150 Pointshere it is
package kayg.funfacts;
import android.graphics.Color; import android.support.v7.app.ActionBarActivity; import android.os.Bundle; import android.util.Log; import android.view.Menu; import android.view.MenuItem; import android.view.View; import android.widget.Button; import android.widget.ExpandableListView; import android.widget.RelativeLayout; import android.widget.TextView; import android.widget.Toast;
import java.util.Random;
public class FunfactsActivity extends ActionBarActivity { public static final String TAG = FunfactsActivity.class.getSimpleName(); private FactBook mFactbook = new FactBook(); private ColorWheel mColorWheel = new ColorWheel();
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_funfacts);
/*declare our view variables and assign them their views*/
final TextView txtfact = (TextView) findViewById(R.id.txtfact);
final Button btnnext = (Button) findViewById(R.id.btnnext);
final RelativeLayout relativeLayout = (RelativeLayout)findViewById(R.id.relativeLayout);
View.OnClickListener listener = new View.OnClickListener() {
@Override
public void onClick(View v) {
String fact = mFactbook.getFact();
int color = mColorWheel.getColor();
relativeLayout.setBackgroundColor(color);
btnnext.setTextColor(color);
//update the label with our dynamic fact
txtfact.setText("");
}
};
btnnext.setOnClickListener(listener);
Toast.makeText(this, "Activity created!", Toast.LENGTH_LONG).show();
Log.d(TAG, "we are logging from the OnCreate() method");
}
}
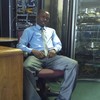
MUZ140698 Kudakwashe Gwaindepi
4,150 Pointsyou'r a genius bro, thank you so much, it just worked
i also figured out that this works also
txtfact.setText(fact); since i had declared my String fact to mFactBook.getFact()

jason phillips
18,131 PointsGlad to see you got it working.