Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial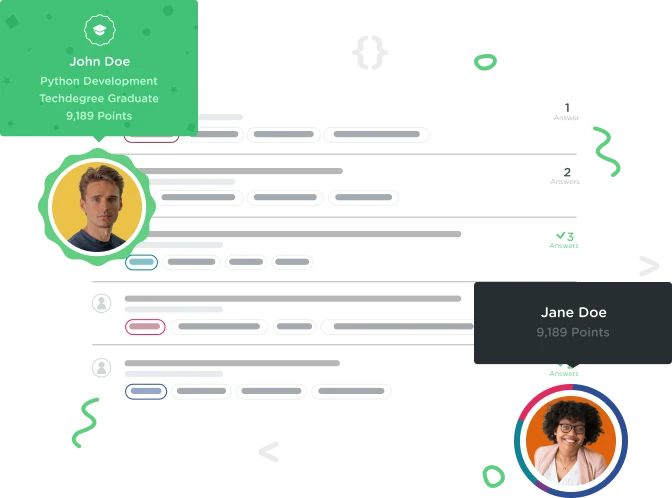

David Hoffman
12,707 PointsI am getting a 404 "Not Found" error in the console. What am I doing wrong?
I am trying to connect to the Treehouse API. I am following the video correctly I think but I am still getting a 404 error. I know that I am missing something small here. Any directional help would be greatly appreciated.
// Problem: We need a simple way to look at a user's badge count and JavaScript points
// Solution: Use Node.js to connect to Treehouse's API to get profile information to print out
//require https module
const https = require('https');
const username = "chalkers";
//Function to print a message to the console
function printMessage(username, badgeCount, points) {
const message = `${username} has ${badgeCount} total badge(s) and ${points} points in JavaScript`;
console.log(message);
}
// Connect to the API url()
const request = https.get('https://teamtreehouse.com/${username}.json', (res) => {
console.log('statusCode: ', res.statusCode);
//read the data
//parse the data
//print the data
});
2 Answers

David Hoffman
12,707 PointsI have answered my own question. Took me a while but I figured out that I was using " ' " in stead of the " ` " in the URL for the GET request.

David Hoffman
12,707 PointsThat is the back-tick character: ` It is located to the left of the "1" key. In ES6 we can use template strings. This allows us to concatenate much easier in JavaScript.
Rodrigo Valladares
9,708 PointsRodrigo Valladares
9,708 Pointshey thanks for post your own question but how do that " ` " what is the keyword ??
Christian Higgins
15,758 PointsChristian Higgins
15,758 PointsFixed my problem too, thanks!