Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial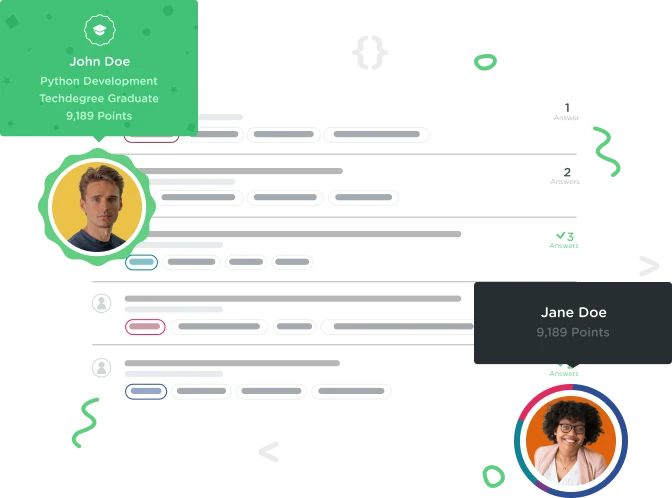

boris said
3,607 PointsI am getting a lot of errors in X-CODE. e.g Extra argument in call.
I am getting errors on my case statements and all of my default statements. Here is my code:
var europeanCapitals: [String] = []
var asianCapitals: [String] = []
var otherCapitals: [String] = []
let world = [
"BEL": "Brussels",
"LIE": "Vaduz",
"BGR": "Sofia",
"USA": "Washington D.C.",
"MEX": "Mexico City",
"BRA": "Brasilia",
"IND": "New Delhi",
"VNM": "Hanoi"]
for (key, value) in world {
switch world {
case "BEL", "LIE", "BGR": europeanCapitals.append("Brussels", "Vaduz", "Sofia")
case "VNM", "IND": asianCapitals.append("New Delhi", "Hanoi")
case "USA", "MEX", "BRA": otherCapitals.append("Washington D.C, "Mexico City", "Brasilia")
default: print("Do nothing")
}
}
[MOD: edited code block. sh]
11 Answers
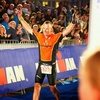
Steve Hunter
57,712 PointsYep. So, again, you want to switch on key
; use switch key
(I've commented your code).
The compiler is saying you can't match a String
, the individual country codes like "BEL" or "IND" , to a String : String
which is what world
is. You need to match a String
to a String
, like key
.
Steve.

boris said
3,607 PointsThank you so much!
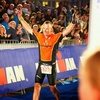
Steve Hunter
57,712 PointsNo problem!
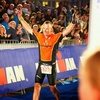
Steve Hunter
57,712 PointsHi Boris,
You're correctly passing the key
and value
into the for
loop.
Try switching on the key
and appending the value
associated with that key
.
So you're going through each key/value pair. At each iteration you are parsing through one key and value. So, switch key
isolates which route your code should take via the case
s you have created. So, append to the appropriate array using case "BEL", "LIE", "BGR": europeanCapitals.append(value)
.
I hope that helps out - I didn't want to just give you the answer as you've done the hard bit already!
Steve.
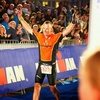
Steve Hunter
57,712 PointsHi Boris,
Can you post your amended code, please?
I could put the solution up, but think there's more learning to be done by going through your code first.
First,each iteration of the for loop deals with one key/value pair. Your switch statement is able to deal with them all. But only one goes through each time the code loops.
You are switching on the key
- that's the "BEL", "IND" bit. You then append the value
of that key
into the array: case "BEL", "LIE", "BGR": europeanCapitals.append(value)
Post your code and let's go through it to reach the solution.
Steve.
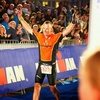
Steve Hunter
57,712 PointsOK. So, you want to switch on key
, not world
. Each key is then tested by your case statements. And amend all your append
method to use value
as a parameter. Your last one doesn't.
And I think that's it!

boris said
3,607 PointsHmmmm, I am still getting the same error. I tried switching the key and value but still the same error. Also, I tried it with just one key and value. I think I understand the error more. I am pretty sure the compiler won't let the array of the capitals be of type String: String which is the type of the array, in order to make it work, I believe I would have to extract the value separately (if at all possible) or change the type to String

boris said
3,607 Pointsfor (key, value) in world {
switch world {
case "BEL", "LIE", "BGR": europeanCapitals.append(value)
case "VNM", "IND": asianCapitals.append(value)
default: otherCapitals.append(world)
}

boris said
3,607 PointsHmmm, I completed that and am still getting errors, could my x-code be out of date?
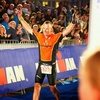
Steve Hunter
57,712 PointsWhat are the errors?

boris said
3,607 PointsExpression pattern of type 'String' cannot match values of type '[String: String]'
This error occurs for each key I try to pass through.
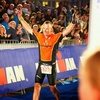
Steve Hunter
57,712 PointsI'll try it in Xcode - did you pass the challenge, though?
Steve.
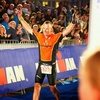
Steve Hunter
57,712 PointsHang on ... that error is saying that you're trying to match a String
to a String : String
.
i.e. you're switching on world
still, which is a String : String
, rather than key
, which is a String
?
Can you paste your code again, please?
The code works fine in Xcode - I think you've still got switch world
?

boris said
3,607 PointsI'll check again

boris said
3,607 PointsNo, it says my code can't be compiled and when I look in preview the error is the same.
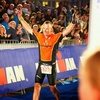
Steve Hunter
57,712 PointsYes, I think because you aren't switching on key
but are still using world
, a String : String
.
You want to use switch key
then the rest is mainly OK.

boris said
3,607 Pointsvar europeanCapitals: [String] = []
var asianCapitals: [String] = []
var otherCapitals: [String] = []
let world = [
"BEL": "Brussels",
"LIE": "Vaduz",
"BGR": "Sofia",
"USA": "Washington D.C.",
"MEX": "Mexico City",
"BRA": "Brasilia",
"IND": "New Delhi",
"VNM": "Hanoi"]
for (key, value) in world {
switch world { // <-- change world to key
case "BEL", "LIE", "BGR": europeanCapitals.append(value)
case "VNM", "IND": asianCapitals.append(value)
default: otherCapitals.append(value)
}
}
boris said
3,607 Pointsboris said
3,607 PointsSorry about my code being compacted. Here is the new version (ignore code from challenge)