Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial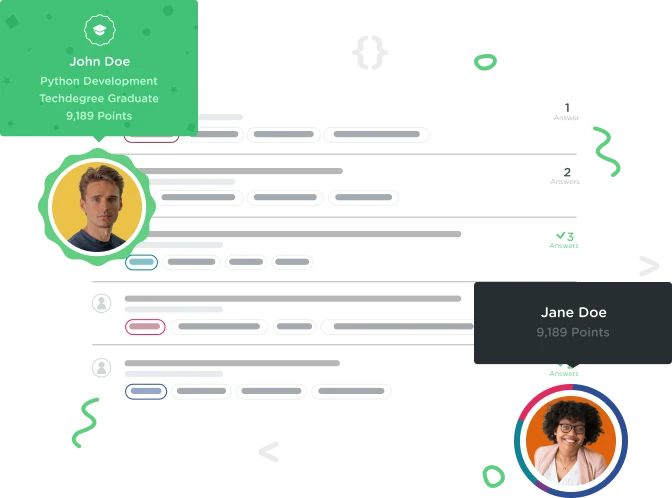

Charles Lambropoulos
Courses Plus Student 2,862 PointsI am getting a TypeError saying "no implicit conversion of String into Integer. Why is an integer required here?
In the contact_list array, it seems the phone number is listed as a string, so I am confused as to why I need to convert it? Or perhaps I am misunderstanding the error message?
contact_list = [
{"name" => "Jason", "phone_number" => "123"},
{"name" => "Nick", "phone_number" => "456"}
]
contact_list.each do |contact|
puts "Contact: #{contact_list["name"]} and Phone Number: #{contact_list["phone_number"]}"
end
1 Answer

Luke Fritz
10,255 PointsThe variables in line 7 should be contact
instead of contact_list
.
contact_list
refers to the Array of Hashes and requires an Integer index, so "name"
is invalid. contact
refers to the current Hash for each iteration.
The following should work.
contact_list = [
{"name" => "Jason", "phone_number" => "123"},
{"name" => "Nick", "phone_number" => "456"}
]
contact_list.each do |contact|
puts "Contact: #{contact["name"]} and Phone Number: #{contact["phone_number"]}"
end
Marcos Rodriguez
6,762 PointsMarcos Rodriguez
6,762 PointsThank you Luke really helped me out. I completely forgot we call each item in the array through the variable contact.