Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial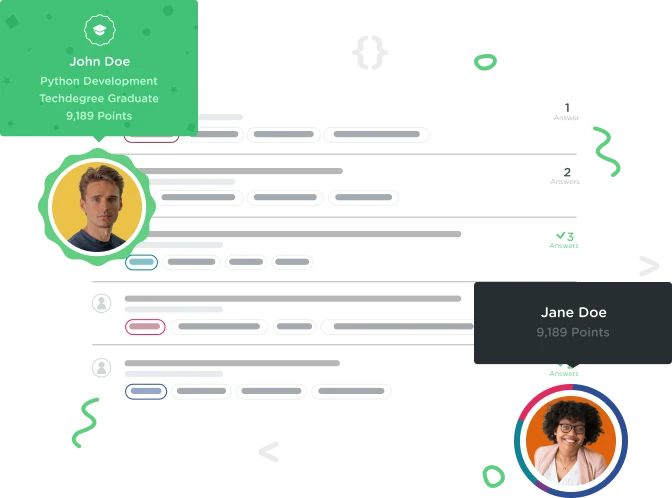
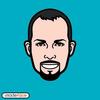
alborz
Full Stack JavaScript Techdegree Graduate 30,885 PointsI am getting an ActionView::Template::Error message after running rspec for adding todo list items
Hi, it looks as if my code is the same as in the video tutorial, however when I run rspec on create_spec.rb I keep getting this error message.
Below are the code from my files.
create_spec.rb:
require 'spec_helper'
describe "Adding todo items" do
let!(:todo_list) {TodoList.create(title: "Grocery list", description: "Groceries")}
def visit_todo_list(list)
visit "/todo_lists"
within "#todo_list_#{list.id}" do
click_link "List Items"
end
end
it "is successful with valid content" do
visit_todo_list(todo_list)
click_link "New Todo Item"
fill_in "Content", with: "Milk"
click_button "Save"
expect(page).to have_content("Added todo list item.")
within("ul.todo_items") do
expect(page).to have_content("Milk")
end
end
end
index_spec.rb:
require 'spec_helper'
describe "Viewing todo items" do
let!(:todo_list) {TodoList.create(title: "Grocery list", description: "Groceries")}
def visit_todo_list(list)
visit "/todo_lists"
within "#todo_list_#{list.id}" do
click_link "List Items"
end
end
it "displays the title of the todo list" do
visit_todo_list(todo_list)
within("h1") do
expect(page).to have_content(todo_list.title)
end
end
it "displays no items when a todo list is empty" do
visit_todo_list(todo_list)
expect(page.all("ul.todo_items li").size).to eq(0)
end
it "displays item content when a todo list has items" do
todo_list.todo_items.create(content: "Milk")
todo_list.todo_items.create(content: "Eggs")
visit_todo_list(todo_list)
expect(page.all("ul.todo_items li").size).to eq(2)
within "ul.todo_items" do
expect(page).to have_content("Milk")
expect(page).to have_content("Eggs")
end
end
end
index.html.erb:
<h1><%= @todo_list.title %></h1>
<ul class="todo_items">
<% @todo_list.todo_items.each do |todo_item| %>
<li><%= todo_item.content %></li>
<% end %>
</ul>
<p>
<%= link_to "New Todo Item", new_todo_list_todo_item_path %>
</p>
todo_items_controller.rb:
class TodoItemsController < ApplicationController
def index
@todo_list = TodoList.find(params[:todo_list_id])
end
def new
@todo_list = TodoList.find(params[:todo_list_id])
@todo_item = @todo_list.todo_items.new
end
def create
@todo_list = TodoList.find(params[:todo_list_id])
@todo_item = @todo_list.todo_items.new(todo_item_params)
if @todo_item.save
flash[:success] = "Added todo list item."
redirect_to todo_list_todo_items_path
else
flash[:error] = "There was a problem adding that todo list item."
render action: :new
end
end
private
def todo_item_params
params[:todo_item].permit(:content)
render action: :new
end
end
new.html.erb:
<%= form_for [@todo_list, @todo_item] do |form| %>
<%= form.label :content %>
<%= form.text_field :content %>
<%= form.submit "Save" %>
<% end %>
application.html.erb:
<!DOCTYPE html>
<html>
<head>
<title>Odot</title>
<%= stylesheet_link_tag 'application', media: 'all', 'data-turbolinks-track' => true %>
<%= javascript_include_tag 'application', 'data-turbolinks-track' => true %>
<%= csrf_meta_tags %>
</head>
<body>
<% flash.each do |type, message| %>
<div class="alert flash <%= type %>">
<%= message %>
</div>
<% end %>
<%= yield %>
</body>
</html>
Here is the test output message:
Adding todo items
is successful with valid content (FAILED - 1)
Failures:
1) Adding todo items is successful with valid content
Failure/Error: click_button "Save"
ActionView::Template::Error:
First argument in form cannot contain nil or be empty
# ./app/views/todo_items/new.html.erb:1:in `_app_views_todo_items_new_html_erb___234205902443850968_70298605578420'
# ./app/controllers/todo_items_controller.rb:26:in `todo_item_params'
# ./app/controllers/todo_items_controller.rb:13:in `create'
# ./spec/features/todo_items/create_spec.rb:17:in `block (2 levels) in <top (required)>'
Thanks!
2 Answers
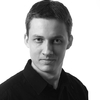
Maciej Czuchnowski
36,441 PointsThis may not be related to the error, but why do you have the render action in this method?
private
def todo_item_params
params[:todo_item].permit(:content)
render action: :new
end
end
This should only contain the permitted params line, nothing more.
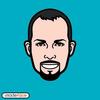
alborz
Full Stack JavaScript Techdegree Graduate 30,885 PointsActually, I removed that and then tested again -- it worked! Must have been clumsily typing it in when going through the tutorial. Thanks!
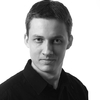
Maciej Czuchnowski
36,441 PointsI'm glad it worked :)