Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial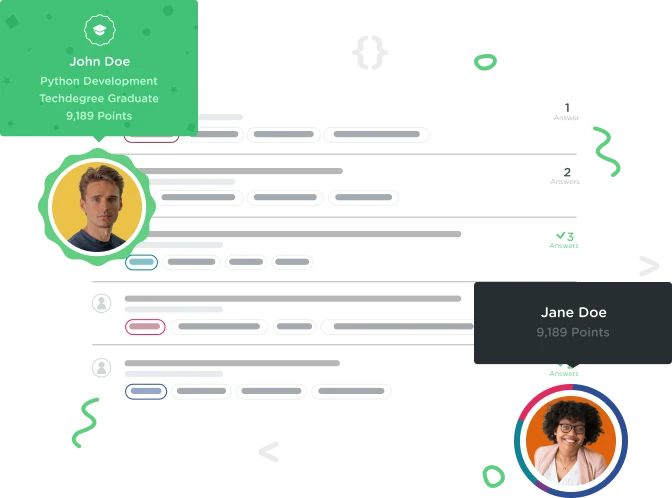

jarrod Reeves
6,357 PointsI am getting an error for my code.
Here is my code for the shirts.php page.
<?php
$products = array();
$products[101] = array(
"name" => "Logo Shirt, Red",
"img" => "img/shirts/shirt-101.jpg",
"price" => 18
);
$products[102] = array(
"name" => "Mike the Frog Shirt, Black",
"img" => "img/shirts/shirt-102.jpg",
"price" => 20
);
$products[103] = array(
"name" => "Mike the Frog Shirt, Blue",
"img" => "img/shirts/shirt-103.jpg",
"price" => 20
);
$products[104] = array(
"name" => "Logo Shirt, Green",
"img" => "img/shirts/shirt-104.jpg",
"price" => 18
);
$products[105] = array(
"name" => "Mike the Frog Shirt, Yellow",
"img" => "img/shirts/shirt-105.jpg",
"price" => 25
);
$products[106] = array(
"name" => "Logo Shirt, Gray",
"img" => "img/shirts/shirt-106.jpg",
"price" => 20
);
$products[107] = array(
"name" => "Logo Shirt, Turquoise",
"img" => "img/shirts/shirt-107.jpg",
"price" => 20
);
$products[108] = array(
"name" => "Logo Shirt, Orange",
"img" => "img/shirts/shirt-108.jpg",
"price" => 25,
);
?>
<?php
$pageTitle = "Mike's Full Catelog of Shirts";
$section = "shirts";
include('inc/header.php'); ?>
<div class="section page">
<div class="wrapper">
<h1>Mikes Full catelog of Shirts </h1>
<ul>
<?php foreach($products as $product){ ?>
<li><?php echo $product;?></li>
<?php };?>
</ul>
</div>
</div>
<?php include('inc/footer.php'); ?>
I am getting the error
Notice: Array to string conversion in C:\xampp\htdocs\shirts.php on line 58
Array
Here is line 58
<li><?php echo $product;?></li>
What am I doing wrong??
4 Answers

Chris Shaw
26,676 PointsHi Jarrod,
Currently you are attempting to echo out an entire array which isn't possible as PHP doesn't have a to_string
method like other languages do, instead you want to be echoing out the <key:value> pairs.
For example
<li><img src="<?php echo $product['img']; ?>" alt=""></li>

justindelamerced
3,032 PointsHi Jarrod,
You can print your array like this, using key=>$value of $products array;
<ul> <?php foreach($products as $key=>$value){ ?> <li><?php echo $value['name'].'<br/>'; echo $value['img'].'<br/>'; echo $value['price']; ?> </li> <?php }; ?> </ul>
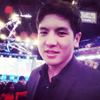
Andrew Zhao
3,267 PointsI am getting the same error. This is confusing because in the video at 2:21, Randy is supposedly using the same code, but he gets something else entirely.
I am using XAMPP on Windows 8. Randy is using MAMP. Perhaps this is a XAMPP vs MAMP issue, where the locals Apache servers are simply processing the PHP differently....

Gavin Ralston
28,770 PointsYou're getting a notice, which is a little like a warning, but it doesn't "break" the script. It just keeps plodding along, although there are server settings which allow you to either ignore them completely, or to generate notices like the one you're seeing so you're more aware of the problem.
Chances are really good in any real world application you wouldn't want to be echoing "Array" to the web page, so you get a notice, followed by the output:
Notice: Array to string conversion in /home/gavin/phptut/shirts.php on line 59
Array
That second line is the same echo'ed text you saw in the video, coupled by the Notice the server generated right before it. So it's "working" just like the video, you're just getting extra output to let you know that you probably didn't want to be doing that..
Chances are good on his development server, he has the flag turned off which generates those Notices.
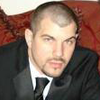
Gary Calhoun
10,317 Pointsthanks I was wondering what was going on with that.