Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial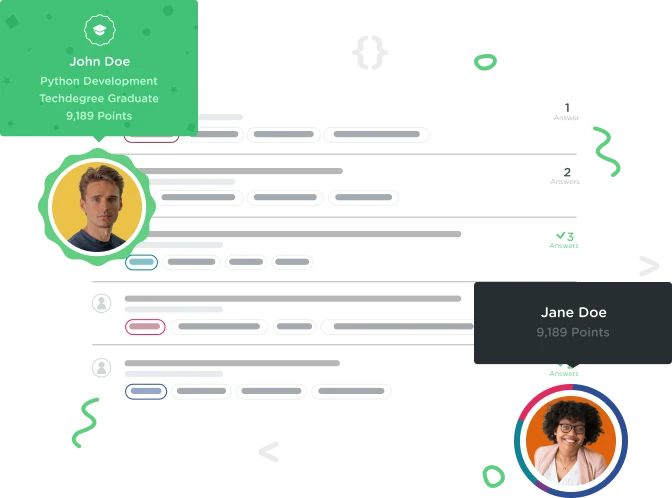

Trey Grider
699 PointsI am getting an error when I try to save an entry, would anyone be available to provide a little guidance?
Receiving this error when I try to save an entry:
Traceback (most recent call last):
File "C:\Users\Trey Grider\Anaconda3\lib\site-packages\peewee.py", line 2936, in execute_sql
cursor.execute(sql, params or ())
sqlite3.IntegrityError: NOT NULL constraint failed: entry.context
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "diary.py", line 62, in <module>
menu_loop()
File "diary.py", line 34, in menu_loop
menu[choice]()
File "diary.py", line 43, in add_entry
Entry.create(content=data)
File "C:\Users\Trey Grider\Anaconda3\lib\site-packages\peewee.py", line 5926, in create
inst.save(force_insert=True)
File "C:\Users\Trey Grider\Anaconda3\lib\site-packages\peewee.py", line 6110, in save
pk_from_cursor = self.insert(**field_dict).execute()
File "C:\Users\Trey Grider\Anaconda3\lib\site-packages\peewee.py", line 1778, in inner
return method(self, database, *args, **kwargs)
File "C:\Users\Trey Grider\Anaconda3\lib\site-packages\peewee.py", line 1849, in execute
return self._execute(database)
File "C:\Users\Trey Grider\Anaconda3\lib\site-packages\peewee.py", line 2567, in _execute
return super(Insert, self)._execute(database)
File "C:\Users\Trey Grider\Anaconda3\lib\site-packages\peewee.py", line 2316, in _execute
cursor = database.execute(self)
File "C:\Users\Trey Grider\Anaconda3\lib\site-packages\peewee.py", line 2949, in execute
return self.execute_sql(sql, params, commit=commit)
File "C:\Users\Trey Grider\Anaconda3\lib\site-packages\peewee.py", line 2943, in execute_sql
self.commit()
File "C:\Users\Trey Grider\Anaconda3\lib\site-packages\peewee.py", line 2725, in __exit__
reraise(new_type, new_type(*exc_args), traceback)
File "C:\Users\Trey Grider\Anaconda3\lib\site-packages\peewee.py", line 183, in reraise
raise value.with_traceback(tb)
File "C:\Users\Trey Grider\Anaconda3\lib\site-packages\peewee.py", line 2936, in execute_sql
cursor.execute(sql, params or ())
peewee.IntegrityError: NOT NULL constraint failed: entry.context
2 Answers

Brandon Galde
12,741 Pointsyou have Entry.create(content=data), however your Entry class does not have content as a parameter (maybe you meant Entry.create(context=data)?)

Aaron Williams
4,313 PointsI had a similar problem, and Brandon's answer is helpful because it would fix the problem.
My issue was that in my Entry Class I had misspelled the attribute variable "content" as "contetnt", you have context as an attribute in the Entry class and content=data below (this is just as Kenneth does in the video).
Even after fixing my typo in he Entry class attribute I also had to delete the diary.db, probably because it was setup with the wrong column in the table.
Trey Grider
699 PointsTrey Grider
699 PointsMy Code:
MOD: I just modified your question and comment to format the output and the code so it's a lot more readable to those who would like to help you out. You can find out how to do this in the Markdown Cheatsheet that's just below the textbox where you type questions or comments. Don't worry, it's easy! :-)