Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial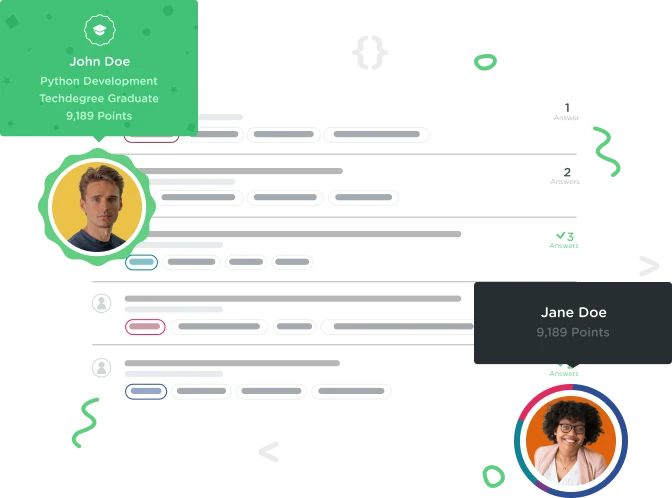

Vaidehi S
5,731 PointsI am getting error when we append checkbox to label.
The code was working fine before these changes and now am getting this error. What is wrong please help?
app.js:55 Uncaught TypeError: Cannot read property 'appendChild' of undefined at createLi (app.js:55) at HTMLFormElement.<anonymous> (app.js:67)
document.addEventListener('DOMContentLoaded', () => {
const form = document.getElementById('registrar');
const input = form.querySelector('input');
const mainDiv =document.querySelector('.main');
const ul = document.getElementById('invitedList');
const div = document.createElement('div');
const filterLabel = document.createElement('label');
const filterCheckBox = document.createElement('input');
filterLabel.textContent = "Hide those who haven't responded";
filterCheckBox.type = 'checkbox';
div.appendChild(filterLabel);
div.appendChild(filterCheckBox);
mainDiv.insertBefore(div, ul);
filterCheckBox.addEventListener('change', (e) => {
const isChecked = e.target.checked;
const lis = ul.children;
if (isChecked) {
for(let i = 0; i < lis.length; i++) {
let li = lis[i];
if(li.className === 'responded') {
li.style.display = '';
} else {
li.style.display = 'none'
}
}
} else {
for(let i = 0; i < lis.length; i++) {
let li = lis[i];
li.style.display = '';
}
}
});
function createLi(text) {
function createElement(elementName, property, value) {
const element = document.createElement(elementName);
element[property] = value;
return element;
}
function appendToLi(elementName, property, value) {
const element = createElement(elementName, property, value);
li.appendChild(element);
};
const li = document.createElement('li');
appendToLi('span', 'textContent', text)
appendToLi('label','textContent','Confirmed').appendChild(createElement('input', 'type', 'checkbox'));
appendToLi('button','textContent', 'edit');
appendToLi('button', 'textContent','remove');
return li;
}
// For Submit button
form.addEventListener('submit', (e) => {
e.preventDefault();
const text = input.value;
input.value = '';
const li = createLi(text)
ul.appendChild(li);
form.reset();
});
//for checkbox
ul.addEventListener('change', (e) => {
const checkbox = e.target;
const checked = checkbox.checked;
const listItem = checkbox.parentNode.parentNode;
if (checked) {
listItem.className = 'responded';
} else {
listItem.className = '';
}
});
//for edit and remove button.
ul.addEventListener('click', (e) => {
if(e.target.tagName === 'BUTTON') {
const button = e.target;
const li = button.parentNode;
const ul = li.parentNode;
if (e.target.textContent === 'Remove') {
ul.removeChild(li);
} else if (button.textContent === 'Edit') {
const span = li.firstElementChild;
const input = document.createElement('input');
input.type = 'text';
input.value = span.textContent;
li.insertBefore(input, span);
li.removeChild(span);
button.textContent = 'Save';
} else if (button.textContent === 'Save') {
const input = li.firstElementChild;
const span = document.createElement('span');
span.textContent = input.value;
li.insertBefore(span, input);
li.removeChild(input);
button.textContent = 'Edit';
}
}
});
});
2 Answers
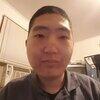
Joseph Yhu
PHP Development Techdegree Graduate 48,637 PointsIt's because your appendToLi()
function isn't returning anything. Making it return the element
variable fixes the problem.

Vaidehi S
5,731 PointsThanks. But Guill in his video also doesnt have return then how did it worked for him.
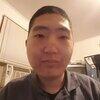
Joseph Yhu
PHP Development Techdegree Graduate 48,637 PointsHe adds the return statement around 9:12 mark.