Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial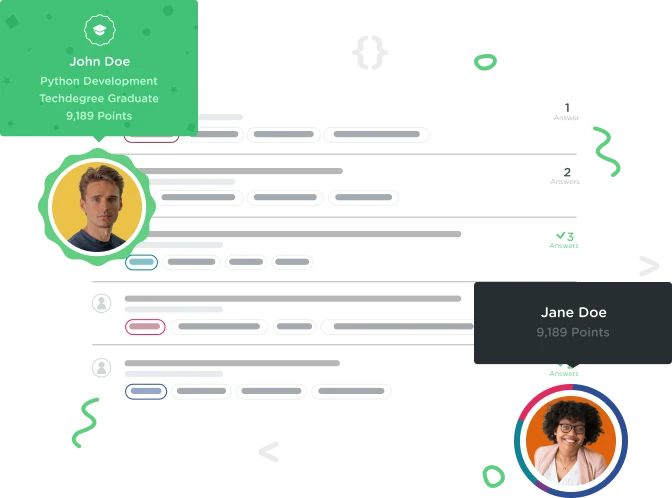
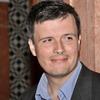
Brian Patterson
19,588 PointsI am getting the error User.create not a function.
When I submit the form I am getting "User.create is not a function". Below is my code.
var express = require('express');
var router = express.Router();
var User = require('../models/user');
//GET /register
router.get('/register', function(req, res, next) {
return res.render('register', { title: 'Sign Up' });
});
//POST /register
router.post('/register', function(req, res, next) {
if (
req.body.email &&
req.body.name &&
req.body.favoriteBook &&
req.body.password &&
req.body.confirmPassword
) {
if (req.body.password != req.body.confirmPassword) {
var err = new Error('Passwords do not match');
err.status = 400;
return next(err);
}
var userData = {
email: req.body.email,
name: req.body.name,
favoriteBook: req.body.favoriteBook,
password: req.body.password
};
//use schema's 'create' method to insert document into Mongo
User.create(userData, function(error, user) {
if (error) {
return next(error);
} else {
return res.redirect('/profile');
}
});
} else {
var err = new Error('All fields required.');
err.status = 400;
return next(err);
}
});
// GET /
router.get('/', function(req, res, next) {
return res.render('index', { title: 'Home' });
});
// GET /about
router.get('/about', function(req, res, next) {
return res.render('about', { title: 'About' });
});
// GET /contact
router.get('/contact', function(req, res, next) {
return res.render('contact', { title: 'Contact' });
});
module.exports = router;
and my app.js file has the following.
var express = require('express');
var bodyParser = require('body-parser');
var mongoose = require('mongoose');
var app = express();
//mongodb connection
mongoose.connect('mongodb://localhost:27017/bookworm');
var db = mongoose.connection;
//mongo error
db.on('error', console.error.bind(console, 'connection error'));
// parse incoming requests
app.use(bodyParser.json());
app.use(bodyParser.urlencoded({ extended: false }));
// serve static files from /public
app.use(express.static(__dirname + '/public'));
// view engine setup
app.set('view engine', 'pug');
app.set('views', __dirname + '/views');
// include routes
var routes = require('./routes/index');
app.use('/', routes);
// catch 404 and forward to error handler
app.use(function(req, res, next) {
var err = new Error('File Not Found');
err.status = 404;
next(err);
});
// error handler
// define as the last app.use callback
app.use(function(err, req, res, next) {
res.status(err.status || 500);
res.render('error', {
message: err.message,
error: {}
});
});
// listen on port 3000
app.listen(3000, function() {
console.log('Express app listening on port 3000');
});
Any help would be appreciated.
1 Answer

Rich Donnellan
Treehouse Moderator 27,696 PointsWhat does the user.js
file look like (var User = require('../models/user');
? Are you pointing to the correct path/filename? Did you npm install mongoose
?
The problem lies somewhere here.
Brian Patterson
19,588 PointsBrian Patterson
19,588 Pointsuser.js
Rich Donnellan
Treehouse Moderator 27,696 PointsRich Donnellan
Treehouse Moderator 27,696 PointsCan you verify you've installed all necessary dependencies?
Brian Patterson
19,588 PointsBrian Patterson
19,588 PointsI think I have just figured it out. I named the Models folder "Model" when it should have been "Models"
Rich Donnellan
Treehouse Moderator 27,696 PointsRich Donnellan
Treehouse Moderator 27,696 PointsAwesome!
It's all in the details! :)