Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial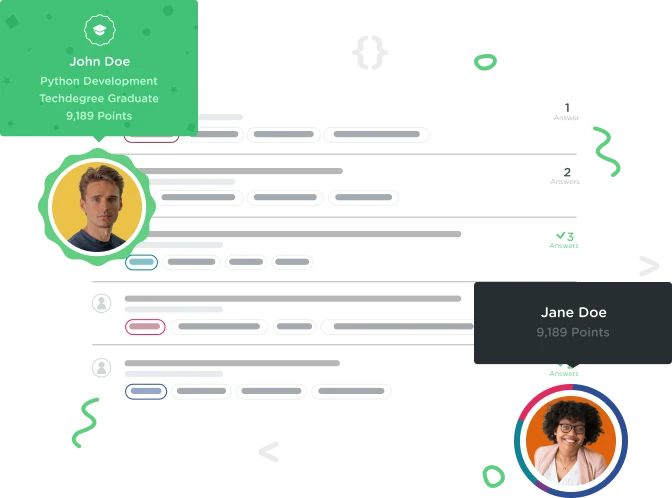
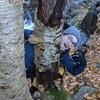
David Richard
4,879 PointsI am getting this error: Strict mode forbids implicit creation of global property 'i'
Can anyone help explain to me why am getting this error or what the correct solution to this assignment might be?
for (i = 5; i <= 100; i++ ) {
console.log(i);
}
2 Answers
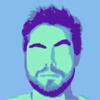
Cameron Childres
11,820 PointsHi David,
You need to initialize the variable i
for your loop with the let
keyword:
for (let i = 5; i <= 100; i++ ) {
console.log(i);
}
The reason for this is that i
doesn't exist yet! If you run this in your browser console it will work, but that's because the interpreter is guessing at what you want to do. The tests here use Strict Mode which forces you to be more explicit with your code:
JavaScript was designed to be easy for novice developers, and sometimes it gives operations which should be errors non-error semantics. Sometimes this fixes the immediate problem, but sometimes this creates worse problems in the future. Strict mode treats these mistakes as errors so that they're discovered and promptly fixed.
When you run your code without strict mode the interpreter assumes "i=5" means:
- make a global variable called
i
and assign it the value5
Writing "let i=5" means:
- make a block-scoped variable called
i
and assign it the value5
The subtle difference here is that in the first example i
will continue to exist outside of the loop. In the second example with the let
keyword it is only used inside the loop and then forgotten about. You can test this out by running this in your browser's console:
for (i=0; i<10; i++) {
console.log(i);
}
console.log(i);
// outside of the loop, i = 10
for (let j=0; j<10; j++) {
console.log(j);
}
console.log(j);
//outside of the loop, j is undefined
Strict mode prevents the "guessing" at your intentions done in the first example so that your variables exist only where you intentionally want them.
If you want more information I'd recommend checking out the following links:
- MDN Documentation on Strict Mode
- MDN Documentation on JavaScript 'for' Statement
- MDN Documentation on JavaScript Block Statements
I hope this helps! Let me know if you have any questions.

John Johnson
11,790 PointsYou must declare i with var, let, or const.